CubbyFlow::Array< T, N > Class Template Reference
#include <Core/Array/Array-Impl.hpp>
Inheritance diagram for CubbyFlow::Array< T, N >:
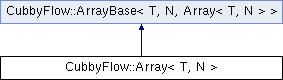
Public Member Functions | |
Array () | |
Array (const Vector< size_t, N > &size, const T &initVal=T{}) | |
template<typename... Args> | |
Array (size_t nx, Args... args) | |
Array (NestedInitializerListsT< T, N > lst) | |
template<typename OtherDerived > | |
Array (const ArrayBase< T, N, OtherDerived > &other) | |
template<typename OtherDerived > | |
Array (const ArrayBase< const T, N, OtherDerived > &other) | |
~Array () override=default | |
Array (const Array &other) | |
Array (Array &&other) noexcept | |
Array & | operator= (const Array &other) |
Array & | operator= (Array &&other) noexcept |
template<typename OtherDerived > | |
Array & | operator= (const ArrayBase< T, N, OtherDerived > &other) |
template<typename OtherDerived > | |
Array & | operator= (const ArrayBase< const T, N, OtherDerived > &other) |
template<typename D > | |
void | CopyFrom (const ArrayBase< T, N, D > &other) |
template<typename D > | |
void | CopyFrom (const ArrayBase< const T, N, D > &other) |
void | Fill (const T &val) |
void | Resize (Vector< size_t, N > size_, const T &initVal=T{}) |
template<typename... Args> | |
void | Resize (size_t nx, Args... args) |
template<size_t M = N> | |
std::enable_if_t<(M==1), void > | Append (const T &val) |
template<typename OtherDerived , size_t M = N> | |
std::enable_if_t<(M==1), void > | Append (const ArrayBase< T, N, OtherDerived > &extra) |
template<typename OtherDerived , size_t M = N> | |
std::enable_if_t<(M==1), void > | Append (const ArrayBase< const T, N, OtherDerived > &extra) |
void | Clear () |
void | Swap (Array &other) |
ArrayView< T, N > | View () |
ArrayView< const T, N > | View () const |
template<typename OtherDerived > | |
Array< T, N > & | operator= (const ArrayBase< T, N, OtherDerived > &other) |
template<typename OtherDerived > | |
Array< T, N > & | operator= (const ArrayBase< const T, N, OtherDerived > &other) |
![]() | |
size_t | Index (size_t i) const |
size_t | Index (size_t i, Args... args) const |
size_t | Index (const Vector< size_t, N > &idx) const |
Pointer | data () |
ConstPointer | data () const |
const Vector< size_t, N > & | Size () const |
std::enable_if_t<(M > 0), size_t > | Width () const |
std::enable_if_t<(M > 0), size_t > | Width () const |
std::enable_if_t<(M > 1), size_t > | Height () const |
std::enable_if_t<(M > 1), size_t > | Height () const |
std::enable_if_t<(M > 2), size_t > | Depth () const |
std::enable_if_t<(M > 2), size_t > | Depth () const |
bool | IsEmpty () const |
size_t | Length () const |
Iterator | begin () |
ConstIterator | begin () const |
Iterator | end () |
ConstIterator | end () const |
Iterator | rbegin () |
ConstIterator | rbegin () const |
Iterator | rend () |
ConstIterator | rend () const |
Reference | At (size_t i) |
ConstReference | At (size_t i) const |
Reference | At (size_t i, Args... args) |
ConstReference | At (size_t i, Args... args) const |
Reference | At (const Vector< size_t, N > &idx) |
ConstReference | At (const Vector< size_t, N > &idx) const |
T & | At (size_t i, Args... args) |
const T & | At (size_t i, Args... args) const |
Reference | operator[] (size_t i) |
ConstReference | operator[] (size_t i) const |
Reference | operator() (size_t i, Args... args) |
ConstReference | operator() (size_t i, Args... args) const |
Reference | operator() (const Vector< size_t, N > &idx) |
ConstReference | operator() (const Vector< size_t, N > &idx) const |
T & | operator() (size_t i, Args... args) |
const T & | operator() (size_t i, Args... args) const |
Additional Inherited Members | |
![]() | |
using | Derived = Array< T, N > |
using | ValueType = T |
using | Reference = T & |
using | ConstReference = const T & |
using | Pointer = T * |
using | ConstPointer = const T * |
using | Iterator = T * |
using | ConstIterator = const T * |
![]() | |
ArrayBase () | |
ArrayBase (const ArrayBase &other) | |
ArrayBase (ArrayBase &&other) noexcept | |
virtual | ~ArrayBase ()=default |
ArrayBase & | operator= (const ArrayBase &other) |
ArrayBase & | operator= (ArrayBase &&other) noexcept |
void | SetPtrAndSize (Pointer ptr, size_t ni, Args... args) |
void | SetPtrAndSize (Pointer data, Vector< size_t, N > size) |
void | SwapPtrAndSize (ArrayBase &other) |
void | ClearPtrAndSize () |
![]() | |
Pointer | m_ptr |
Vector< size_t, N > | m_size |
Constructor & Destructor Documentation
◆ Array() [1/8]
template<typename T , size_t N>
CubbyFlow::Array< T, N >::Array | ( | ) |
◆ Array() [2/8]
template<typename T, size_t N>
CubbyFlow::Array< T, N >::Array | ( | const Vector< size_t, N > & | size, |
const T & | initVal = T{} |
||
) |
◆ Array() [3/8]
template<typename T , size_t N>
template<typename... Args>
CubbyFlow::Array< T, N >::Array | ( | size_t | nx, |
Args... | args | ||
) |
◆ Array() [4/8]
template<typename T, size_t N>
CubbyFlow::Array< T, N >::Array | ( | NestedInitializerListsT< T, N > | lst | ) |
◆ Array() [5/8]
template<typename T, size_t N>
template<typename OtherDerived>
CubbyFlow::Array< T, N >::Array | ( | const ArrayBase< T, N, OtherDerived > & | other | ) |
◆ Array() [6/8]
template<typename T, size_t N>
template<typename OtherDerived>
CubbyFlow::Array< T, N >::Array | ( | const ArrayBase< const T, N, OtherDerived > & | other | ) |
◆ ~Array()
template<typename T, size_t N>
|
overridedefault |
◆ Array() [7/8]
template<typename T, size_t N>
CubbyFlow::Array< T, N >::Array | ( | const Array< T, N > & | other | ) |
◆ Array() [8/8]
template<typename T, size_t N>
|
noexcept |
Member Function Documentation
◆ Append() [1/3]
template<typename T, size_t N>
template<size_t M>
std::enable_if_t<(M==1), void > CubbyFlow::Array< T, N >::Append | ( | const T & | val | ) |
◆ Append() [2/3]
template<typename T, size_t N>
template<typename OtherDerived , size_t M>
std::enable_if_t<(M==1), void > CubbyFlow::Array< T, N >::Append | ( | const ArrayBase< T, N, OtherDerived > & | extra | ) |
◆ Append() [3/3]
template<typename T, size_t N>
template<typename OtherDerived , size_t M>
std::enable_if_t<(M==1), void > CubbyFlow::Array< T, N >::Append | ( | const ArrayBase< const T, N, OtherDerived > & | extra | ) |
◆ Clear()
template<typename T , size_t N>
void CubbyFlow::Array< T, N >::Clear | ( | ) |
◆ CopyFrom() [1/2]
template<typename T, size_t N>
template<typename D >
void CubbyFlow::Array< T, N >::CopyFrom | ( | const ArrayBase< T, N, D > & | other | ) |
◆ CopyFrom() [2/2]
template<typename T, size_t N>
template<typename D >
void CubbyFlow::Array< T, N >::CopyFrom | ( | const ArrayBase< const T, N, D > & | other | ) |
◆ Fill()
template<typename T, size_t N>
void CubbyFlow::Array< T, N >::Fill | ( | const T & | val | ) |
◆ operator=() [1/6]
template<typename T , size_t N>
Array< T, N > & CubbyFlow::Array< T, N >::operator= | ( | const Array< T, N > & | other | ) |
◆ operator=() [2/6]
template<typename T , size_t N>
|
noexcept |
◆ operator=() [3/6]
template<typename T, size_t N>
template<typename OtherDerived >
Array& CubbyFlow::Array< T, N >::operator= | ( | const ArrayBase< T, N, OtherDerived > & | other | ) |
◆ operator=() [4/6]
template<typename T, size_t N>
template<typename OtherDerived >
Array& CubbyFlow::Array< T, N >::operator= | ( | const ArrayBase< const T, N, OtherDerived > & | other | ) |
◆ operator=() [5/6]
template<typename T, size_t N>
template<typename OtherDerived >
Array<T, N>& CubbyFlow::Array< T, N >::operator= | ( | const ArrayBase< T, N, OtherDerived > & | other | ) |
◆ operator=() [6/6]
template<typename T, size_t N>
template<typename OtherDerived >
Array<T, N>& CubbyFlow::Array< T, N >::operator= | ( | const ArrayBase< const T, N, OtherDerived > & | other | ) |
◆ Resize() [1/2]
template<typename T, size_t N>
void CubbyFlow::Array< T, N >::Resize | ( | Vector< size_t, N > | size_, |
const T & | initVal = T{} |
||
) |
◆ Resize() [2/2]
template<typename T , size_t N>
template<typename... Args>
void CubbyFlow::Array< T, N >::Resize | ( | size_t | nx, |
Args... | args | ||
) |
◆ Swap()
template<typename T , size_t N>
void CubbyFlow::Array< T, N >::Swap | ( | Array< T, N > & | other | ) |
◆ View() [1/2]
template<typename T , size_t N>
ArrayView< T, N > CubbyFlow::Array< T, N >::View | ( | ) |
◆ View() [2/2]
template<typename T , size_t N>
ArrayView< const T, N > CubbyFlow::Array< T, N >::View | ( | ) | const |
The documentation for this class was generated from the following files:
- Core/Array/Array-Impl.hpp
- Core/Array/Array.hpp