N-D box geometry. More...
#include <Core/Geometry/Box.hpp>
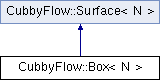
Classes | |
class | Builder |
Front-end to create Box objects step by step. More... | |
Public Member Functions | |
Box (const Transform< N > &_transform=Transform< N >{}, bool _isNormalFlipped=false) | |
Constructs (0, 0, ...) x (1, 1, ...) box. More... | |
Box (const Vector< double, N > &lowerCorner, const Vector< double, N > &upperCorner, const Transform< N > &_transform=Transform< N >{}, bool _isNormalFlipped=false) | |
Constructs a box with given lowerCorner and upperCorner . More... | |
Box (const BoundingBox< double, N > &boundingBox, const Transform< N > &_transform=Transform< N >{}, bool _isNormalFlipped=false) | |
Constructs a box with BoundingBox instance. More... | |
~Box () override=default | |
Default virtual destructor. More... | |
Box (const Box &other) | |
Copy constructor. More... | |
Box (Box &&other) noexcept | |
Move constructor. More... | |
Box & | operator= (const Box &other) |
Copy assignment operator. More... | |
Box & | operator= (Box &&other) noexcept |
Move assignment operator. More... | |
![]() | |
Surface (const Transform< N > &transform=Transform< N >(), bool isNormalFlipped=false) | |
Constructs a surface with normal direction. More... | |
virtual | ~Surface ()=default |
Default virtual destructor. More... | |
Surface (const Surface &other) | |
Copy constructor. More... | |
Surface (Surface &&other) noexcept | |
Move constructor. More... | |
Surface & | operator= (const Surface &other) |
Copy assignment operator. More... | |
Surface & | operator= (Surface &&other) noexcept |
Move assignment operator. More... | |
Vector< double, N > | ClosestPoint (const Vector< double, N > &otherPoint) const |
BoundingBox< double, N > | GetBoundingBox () const |
Returns the bounding box of this surface object. More... | |
bool | Intersects (const Ray< double, N > &ray) const |
Returns true if the given ray intersects with this surface object. More... | |
double | ClosestDistance (const Vector< double, N > &otherPoint) const |
SurfaceRayIntersection< N > | ClosestIntersection (const Ray< double, N > &ray) const |
Returns the closest intersection point for given ray . More... | |
Vector< double, N > | ClosestNormal (const Vector< double, N > &otherPoint) const |
virtual void | UpdateQueryEngine () |
Updates internal spatial query engine. More... | |
virtual bool | IsBounded () const |
Returns true if bounding box can be defined. More... | |
virtual bool | IsValidGeometry () const |
Returns true if the surface is a valid geometry. More... | |
bool | IsInside (const Vector< double, N > &otherPoint) const |
Static Public Member Functions | |
static Builder | GetBuilder () |
Returns builder fox Box. More... | |
Public Attributes | |
BoundingBox< double, N > | bound |
Bounding box of this box. More... | |
![]() | |
Transform< N > | transform |
Local-to-world transform. More... | |
bool | isNormalFlipped = false |
Flips normal. More... | |
Protected Member Functions | |
Vector< double, N > | ClosestPointLocal (const Vector< double, N > &otherPoint) const override |
bool | IntersectsLocal (const Ray< double, N > &ray) const override |
BoundingBox< double, N > | BoundingBoxLocal () const override |
Returns the bounding box of this surface object in local frame. More... | |
Vector< double, N > | ClosestNormalLocal (const Vector< double, N > &otherPoint) const override |
SurfaceRayIntersection< N > | ClosestIntersectionLocal (const Ray< double, N > &ray) const override |
Returns the closest intersection point for given ray in local frame. More... | |
![]() | |
virtual double | ClosestDistanceLocal (const Vector< double, N > &otherPoint) const |
virtual bool | IsInsideLocal (const Vector< double, N > &otherPoint) const |
Detailed Description
template<size_t N>
class CubbyFlow::Box< N >
N-D box geometry.
This class represents N-D box geometry which extends Surface class by overriding surface-related queries. This box implementation is an axis-aligned box that wraps lower-level primitive type, BoundingBox.
Constructor & Destructor Documentation
◆ Box() [1/5]
CubbyFlow::Box< N >::Box | ( | const Transform< N > & | _transform = Transform< N >{} , |
bool | _isNormalFlipped = false |
||
) |
Constructs (0, 0, ...) x (1, 1, ...) box.
◆ Box() [2/5]
CubbyFlow::Box< N >::Box | ( | const Vector< double, N > & | lowerCorner, |
const Vector< double, N > & | upperCorner, | ||
const Transform< N > & | _transform = Transform< N >{} , |
||
bool | _isNormalFlipped = false |
||
) |
Constructs a box with given lowerCorner
and upperCorner
.
◆ Box() [3/5]
CubbyFlow::Box< N >::Box | ( | const BoundingBox< double, N > & | boundingBox, |
const Transform< N > & | _transform = Transform< N >{} , |
||
bool | _isNormalFlipped = false |
||
) |
Constructs a box with BoundingBox instance.
◆ ~Box()
|
overridedefault |
Default virtual destructor.
◆ Box() [4/5]
CubbyFlow::Box< N >::Box | ( | const Box< N > & | other | ) |
Copy constructor.
◆ Box() [5/5]
|
noexcept |
Move constructor.
Member Function Documentation
◆ BoundingBoxLocal()
|
overrideprotectedvirtual |
Returns the bounding box of this surface object in local frame.
Implements CubbyFlow::Surface< N >.
◆ ClosestIntersectionLocal()
|
overrideprotectedvirtual |
Returns the closest intersection point for given ray
in local frame.
Implements CubbyFlow::Surface< N >.
◆ ClosestNormalLocal()
|
overrideprotectedvirtual |
Returns the normal to the closest point on the surface from the given point otherPoint
in local frame.
Implements CubbyFlow::Surface< N >.
◆ ClosestPointLocal()
|
overrideprotectedvirtual |
Returns the closest point from the given point otherPoint
to the surface in local frame.
Implements CubbyFlow::Surface< N >.
◆ GetBuilder()
|
static |
Returns builder fox Box.
◆ IntersectsLocal()
|
overrideprotectedvirtual |
Returns true if the given ray
intersects with this surface object in local frame.
Reimplemented from CubbyFlow::Surface< N >.
◆ operator=() [1/2]
Box& CubbyFlow::Box< N >::operator= | ( | const Box< N > & | other | ) |
Copy assignment operator.
◆ operator=() [2/2]
|
noexcept |
Move assignment operator.
Member Data Documentation
◆ bound
BoundingBox<double, N> CubbyFlow::Box< N >::bound |
Bounding box of this box.
The documentation for this class was generated from the following file:
- Core/Geometry/Box.hpp