2-D Particle-in-Cell (PIC) implementation. More...
#include <Core/Solver/Hybrid/PIC/PICSolver2.hpp>
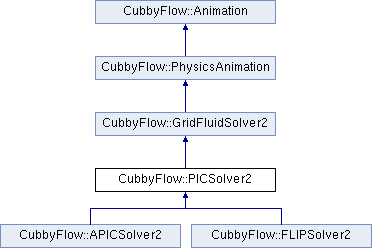
Classes | |
class | Builder |
Front-end to create PICSolver2 objects step by step. More... | |
Public Member Functions | |
PICSolver2 () | |
Default constructor. More... | |
PICSolver2 (const Vector2UZ &resolution, const Vector2D &gridSpacing, const Vector2D &gridOrigin) | |
Constructs solver with initial grid size. More... | |
PICSolver2 (const PICSolver2 &)=delete | |
Deleted copy constructor. More... | |
PICSolver2 (PICSolver2 &&) noexcept=delete | |
Deleted move constructor. More... | |
~PICSolver2 () override=default | |
Default virtual destructor. More... | |
PICSolver2 & | operator= (const PICSolver2 &)=delete |
Deleted copy assignment operator. More... | |
PICSolver2 & | operator= (PICSolver2 &&) noexcept=delete |
Deleted move assignment operator. More... | |
ScalarGrid2Ptr | GetSignedDistanceField () const |
Returns the signed-distance field of particles. More... | |
const ParticleSystemData2Ptr & | GetParticleSystemData () const |
Returns the particle system data. More... | |
const ParticleEmitter2Ptr & | GetParticleEmitter () const |
Returns the particle emitter. More... | |
void | SetParticleEmitter (const ParticleEmitter2Ptr &newEmitter) |
Sets the particle emitter. More... | |
![]() | |
GridFluidSolver2 () | |
Default constructor. More... | |
GridFluidSolver2 (const Vector2UZ &resolution, const Vector2D &gridSpacing, const Vector2D &gridOrigin) | |
Constructs solver with initial grid size. More... | |
GridFluidSolver2 (const GridFluidSolver2 &)=delete | |
Deleted copy constructor. More... | |
GridFluidSolver2 (GridFluidSolver2 &&) noexcept=delete | |
Deleted move constructor. More... | |
~GridFluidSolver2 () override=default | |
Default virtual destructor. More... | |
GridFluidSolver2 & | operator= (const GridFluidSolver2 &)=delete |
Deleted copy assignment operator. More... | |
GridFluidSolver2 & | operator= (GridFluidSolver2 &&) noexcept=delete |
Deleted move assignment operator. More... | |
const Vector2D & | GetGravity () const |
Returns the gravity vector of the system. More... | |
void | SetGravity (const Vector2D &newGravity) |
Sets the gravity of the system. More... | |
double | GetViscosityCoefficient () const |
Returns the viscosity coefficient. More... | |
void | SetViscosityCoefficient (double newValue) |
Sets the viscosity coefficient. More... | |
double | GetCFL (double timeIntervalInSeconds) const |
Returns the CFL number from the current velocity field for given time interval. More... | |
double | GetMaxCFL () const |
Returns the max allowed CFL number. More... | |
void | SetMaxCFL (double newCFL) |
Sets the max allowed CFL number. More... | |
bool | GetUseCompressedLinearSystem () const |
Returns true if the solver is using compressed linear system. More... | |
void | SetUseCompressedLinearSystem (bool isOn) |
Sets whether the solver should use compressed linear system. More... | |
const AdvectionSolver2Ptr & | GetAdvectionSolver () const |
Returns the advection solver instance. More... | |
void | SetAdvectionSolver (const AdvectionSolver2Ptr &newSolver) |
Sets the advection solver. More... | |
const GridDiffusionSolver2Ptr & | GetDiffusionSolver () const |
Returns the diffusion solver instance. More... | |
void | SetDiffusionSolver (const GridDiffusionSolver2Ptr &newSolver) |
Sets the diffusion solver. More... | |
const GridPressureSolver2Ptr & | GetPressureSolver () const |
Returns the pressure solver instance. More... | |
void | SetPressureSolver (const GridPressureSolver2Ptr &newSolver) |
Sets the pressure solver. More... | |
int | GetClosedDomainBoundaryFlag () const |
Returns the closed domain boundary flag. More... | |
void | SetClosedDomainBoundaryFlag (int flag) |
Sets the closed domain boundary flag. More... | |
const GridSystemData2Ptr & | GetGridSystemData () const |
Returns the grid system data. More... | |
void | ResizeGrid (const Vector2UZ &newSize, const Vector2D &newGridSpacing, const Vector2D &newGridOrigin) const |
Resizes grid system data. More... | |
Vector2UZ | GetResolution () const |
Returns the resolution of the grid system data. More... | |
Vector2D | GetGridSpacing () const |
Returns the grid spacing of the grid system data. More... | |
Vector2D | GetGridOrigin () const |
Returns the origin of the grid system data. More... | |
const FaceCenteredGrid2Ptr & | GetVelocity () const |
Returns the velocity field. More... | |
const Collider2Ptr & | GetCollider () const |
Returns the collider. More... | |
void | SetCollider (const Collider2Ptr &newCollider) |
Sets the collider. More... | |
const GridEmitter2Ptr & | GetEmitter () const |
Returns the emitter. More... | |
void | SetEmitter (const GridEmitter2Ptr &newEmitter) |
Sets the emitter. More... | |
![]() | |
PhysicsAnimation () | |
Default constructor. More... | |
PhysicsAnimation (const PhysicsAnimation &)=default | |
Default copy constructor. More... | |
PhysicsAnimation (PhysicsAnimation &&) noexcept=default | |
Default move constructor. More... | |
virtual | ~PhysicsAnimation ()=default |
Default virtual destructor. More... | |
PhysicsAnimation & | operator= (const PhysicsAnimation &)=default |
Default copy assignment operator. More... | |
PhysicsAnimation & | operator= (PhysicsAnimation &&) noexcept=default |
Default move assignment operator. More... | |
bool | GetIsUsingFixedSubTimeSteps () const |
Returns true if fixed sub-timestepping is used. More... | |
void | SetIsUsingFixedSubTimeSteps (bool isUsing) |
Sets true if fixed sub-timestepping is used. More... | |
unsigned int | GetNumberOfFixedSubTimeSteps () const |
Returns the number of fixed sub-timesteps. More... | |
void | SetNumberOfFixedSubTimeSteps (unsigned int numberOfSteps) |
Sets the number of fixed sub-timesteps. More... | |
void | AdvanceSingleFrame () |
Advances a single frame. More... | |
Frame | GetCurrentFrame () const |
Returns current frame. More... | |
void | SetCurrentFrame (const Frame &frame) |
Sets current frame cursor (but do not invoke update()). More... | |
double | GetCurrentTimeInSeconds () const |
Returns current time in seconds. More... | |
![]() | |
Animation ()=default | |
Default constructor. More... | |
Animation (const Animation &)=default | |
Default copy constructor. More... | |
Animation (Animation &&) noexcept=default | |
Default move constructor. More... | |
virtual | ~Animation ()=default |
Default virtual destructor. More... | |
Animation & | operator= (const Animation &)=default |
Default copy assignment operator. More... | |
Animation & | operator= (Animation &&) noexcept=default |
Default move assignment operator. More... | |
void | Update (const Frame &frame) |
Updates animation state for given frame . More... | |
Static Public Member Functions | |
static Builder | GetBuilder () |
Returns builder fox PICSolver2. More... | |
![]() | |
static Builder | GetBuilder () |
Returns builder fox GridFluidSolver2. More... | |
Protected Member Functions | |
void | OnInitialize () override |
Initializes the simulator. More... | |
void | OnBeginAdvanceTimeStep (double timeIntervalInSeconds) override |
Invoked before a simulation time-step begins. More... | |
void | ComputeAdvection (double timeIntervalInSeconds) override |
Computes the advection term of the fluid solver. More... | |
ScalarField2Ptr | GetFluidSDF () const override |
Returns the signed-distance field of the fluid. More... | |
virtual void | TransferFromParticlesToGrids () |
Transfers velocity field from particles to grids. More... | |
virtual void | TransferFromGridsToParticles () |
Transfers velocity field from grids to particles. More... | |
virtual void | MoveParticles (double timeIntervalInSeconds) |
Moves particles. More... | |
![]() | |
void | OnAdvanceTimeStep (double timeIntervalInSeconds) override |
Called when advancing a single time-step. More... | |
unsigned int | GetNumberOfSubTimeSteps (double timeIntervalInSeconds) const override |
Returns the required sub-time-steps for given time interval. More... | |
virtual void | OnEndAdvanceTimeStep (double timeIntervalInSeconds) |
Called at the end of a time-step. More... | |
virtual void | ComputeExternalForces (double timeIntervalInSeconds) |
Computes the external force terms. More... | |
virtual void | ComputeViscosity (double timeIntervalInSeconds) |
Computes the viscosity term using the diffusion solver. More... | |
virtual void | ComputePressure (double timeIntervalInSeconds) |
Computes the pressure term using the pressure solver. More... | |
void | ComputeGravity (double timeIntervalInSeconds) |
Computes the gravity term. More... | |
void | ApplyBoundaryCondition () const |
Applies the boundary condition to the velocity field. More... | |
void | ExtrapolateIntoCollider (ScalarGrid2 *grid) |
Extrapolates given field into the collider-occupied region. More... | |
void | ExtrapolateIntoCollider (CollocatedVectorGrid2 *grid) |
Extrapolates given field into the collider-occupied region. More... | |
void | ExtrapolateIntoCollider (FaceCenteredGrid2 *grid) |
Extrapolates given field into the collider-occupied region. More... | |
ScalarField2Ptr | GetColliderSDF () const |
Returns the signed-distance field representation of the collider. More... | |
VectorField2Ptr | GetColliderVelocityField () const |
Returns the velocity field of the collider. More... | |
Protected Attributes | |
Array2< char > | m_uMarkers |
Array2< char > | m_vMarkers |
Detailed Description
2-D Particle-in-Cell (PIC) implementation.
This class implements 2-D Particle-in-Cell (PIC) method by inheriting GridFluidSolver2. Since it is a grid-particle hybrid method, the solver also has a particle system to track fluid particles.
- See also
- Zhu, Yongning, and Robert Bridson. "Animating sand as a fluid." ACM Transactions on Graphics (TOG). Vol. 24. No. 3. ACM, 2005.
Constructor & Destructor Documentation
◆ PICSolver2() [1/4]
CubbyFlow::PICSolver2::PICSolver2 | ( | ) |
Default constructor.
◆ PICSolver2() [2/4]
CubbyFlow::PICSolver2::PICSolver2 | ( | const Vector2UZ & | resolution, |
const Vector2D & | gridSpacing, | ||
const Vector2D & | gridOrigin | ||
) |
Constructs solver with initial grid size.
◆ PICSolver2() [3/4]
|
delete |
Deleted copy constructor.
◆ PICSolver2() [4/4]
|
deletenoexcept |
Deleted move constructor.
◆ ~PICSolver2()
|
overridedefault |
Default virtual destructor.
Member Function Documentation
◆ ComputeAdvection()
|
overrideprotectedvirtual |
Computes the advection term of the fluid solver.
Reimplemented from CubbyFlow::GridFluidSolver2.
◆ GetBuilder()
|
static |
Returns builder fox PICSolver2.
◆ GetFluidSDF()
|
overrideprotectedvirtual |
Returns the signed-distance field of the fluid.
Reimplemented from CubbyFlow::GridFluidSolver2.
◆ GetParticleEmitter()
const ParticleEmitter2Ptr& CubbyFlow::PICSolver2::GetParticleEmitter | ( | ) | const |
Returns the particle emitter.
◆ GetParticleSystemData()
const ParticleSystemData2Ptr& CubbyFlow::PICSolver2::GetParticleSystemData | ( | ) | const |
Returns the particle system data.
◆ GetSignedDistanceField()
ScalarGrid2Ptr CubbyFlow::PICSolver2::GetSignedDistanceField | ( | ) | const |
Returns the signed-distance field of particles.
◆ MoveParticles()
|
protectedvirtual |
Moves particles.
◆ OnBeginAdvanceTimeStep()
|
overrideprotectedvirtual |
Invoked before a simulation time-step begins.
Reimplemented from CubbyFlow::GridFluidSolver2.
◆ OnInitialize()
|
overrideprotectedvirtual |
Initializes the simulator.
Reimplemented from CubbyFlow::GridFluidSolver2.
◆ operator=() [1/2]
|
delete |
Deleted copy assignment operator.
◆ operator=() [2/2]
|
deletenoexcept |
Deleted move assignment operator.
◆ SetParticleEmitter()
void CubbyFlow::PICSolver2::SetParticleEmitter | ( | const ParticleEmitter2Ptr & | newEmitter | ) |
Sets the particle emitter.
◆ TransferFromGridsToParticles()
|
protectedvirtual |
Transfers velocity field from grids to particles.
Reimplemented in CubbyFlow::FLIPSolver2, and CubbyFlow::APICSolver2.
◆ TransferFromParticlesToGrids()
|
protectedvirtual |
Transfers velocity field from particles to grids.
Reimplemented in CubbyFlow::FLIPSolver2, and CubbyFlow::APICSolver2.
Member Data Documentation
◆ m_uMarkers
|
protected |
◆ m_vMarkers
|
protected |
The documentation for this class was generated from the following file:
- Core/Solver/Hybrid/PIC/PICSolver2.hpp