N-D particle system data. More...
#include <Core/Particle/ParticleSystemData.hpp>
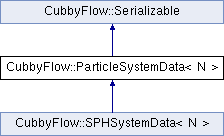
Public Types | |
using | ScalarData = Array1< double > |
Scalar data chunk. More... | |
using | VectorData = Array1< Vector< double, N > > |
Vector data chunk. More... | |
Public Member Functions | |
ParticleSystemData () | |
Default constructor. More... | |
ParticleSystemData (size_t numberOfParticles) | |
Constructs particle system data with given number of particles. More... | |
~ParticleSystemData () override=default | |
Default virtual destructor. More... | |
ParticleSystemData (const ParticleSystemData &other) | |
Copy constructor. More... | |
ParticleSystemData (ParticleSystemData &&other) noexcept | |
Move constructor. More... | |
ParticleSystemData & | operator= (const ParticleSystemData &other) |
Copy assignment operator. More... | |
ParticleSystemData & | operator= (ParticleSystemData &&other) noexcept |
Move assignment operator. More... | |
void | Resize (size_t newNumberOfParticles) |
Resizes the number of particles of the container. More... | |
size_t | NumberOfParticles () const |
Returns the number of particles. More... | |
size_t | AddScalarData (double initialVal=0.0) |
Adds a scalar data layer and returns its index. More... | |
size_t | AddVectorData (const Vector< double, N > &initialVal=Vector< double, N >{}) |
Adds a vector data layer and returns its index. More... | |
double | Radius () const |
Returns the radius of the particles. More... | |
virtual void | SetRadius (double newRadius) |
Sets the radius of the particles. More... | |
double | Mass () const |
Returns the mass of the particles. More... | |
virtual void | SetMass (double newMass) |
Sets the mass of the particles. More... | |
ConstArrayView1< Vector< double, N > > | Positions () const |
Returns the position array (immutable). More... | |
ArrayView1< Vector< double, N > > | Positions () |
Returns the position array (mutable). More... | |
ConstArrayView1< Vector< double, N > > | Velocities () const |
Returns the velocity array (immutable). More... | |
ArrayView1< Vector< double, N > > | Velocities () |
Returns the velocity array (mutable). More... | |
ConstArrayView1< Vector< double, N > > | Forces () const |
Returns the force array (immutable). More... | |
ArrayView1< Vector< double, N > > | Forces () |
Returns the force array (mutable). More... | |
ConstArrayView1< double > | ScalarDataAt (size_t idx) const |
Returns custom scalar data layer at given index (immutable). More... | |
ArrayView1< double > | ScalarDataAt (size_t idx) |
Returns custom scalar data layer at given index (mutable). More... | |
ConstArrayView1< Vector< double, N > > | VectorDataAt (size_t idx) const |
Returns custom vector data layer at given index (immutable). More... | |
ArrayView1< Vector< double, N > > | VectorDataAt (size_t idx) |
Returns custom vector data layer at given index (mutable). More... | |
void | AddParticle (const Vector< double, N > &newPosition, const Vector< double, N > &newVelocity=Vector< double, N >(), const Vector< double, N > &newForce=Vector< double, N >()) |
Adds a particle to the data structure. More... | |
void | AddParticles (const ConstArrayView1< Vector< double, N >> &newPositions, const ConstArrayView1< Vector< double, N >> &newVelocities=ConstArrayView1< Vector< double, N >>(), const ConstArrayView1< Vector< double, N >> &newForces=ConstArrayView1< Vector< double, N >>()) |
Adds particles to the data structure. More... | |
const std::shared_ptr< PointNeighborSearcher< N > > & | NeighborSearcher () const |
Returns neighbor searcher. More... | |
void | SetNeighborSearcher (const std::shared_ptr< PointNeighborSearcher< N >> &newNeighborSearcher) |
Sets neighbor searcher. More... | |
const Array1< Array1< size_t > > & | NeighborLists () const |
Returns neighbor lists. More... | |
void | BuildNeighborSearcher (double maxSearchRadius) |
Builds neighbor searcher with given search radius. More... | |
void | BuildNeighborLists (double maxSearchRadius) |
Builds neighbor lists with given search radius. More... | |
void | Serialize (std::vector< uint8_t > *buffer) const override |
Serializes this particle system data to the buffer. More... | |
void | Deserialize (const std::vector< uint8_t > &buffer) override |
Deserializes this particle system data from the buffer. More... | |
void | Set (const ParticleSystemData &other) |
Copies from other particle system data. More... | |
![]() | |
Serializable ()=default | |
Default constructor. More... | |
Serializable (const Serializable &)=default | |
Default copy constructor. More... | |
Serializable (Serializable &&) noexcept=default | |
Default move constructor. More... | |
virtual | ~Serializable ()=default |
Default virtual destructor. More... | |
Serializable & | operator= (const Serializable &)=default |
Default copy assignment operator. More... | |
Serializable & | operator= (Serializable &&) noexcept=default |
Default move assignment operator. More... | |
Static Protected Member Functions | |
template<size_t M = N> | |
static std::enable_if_t< M==2, void > | Serialize (const ParticleSystemData< 2 > &particles, flatbuffers::FlatBufferBuilder *builder, flatbuffers::Offset< fbs::ParticleSystemData2 > *fbsParticleSystemData) |
template<size_t M = N> | |
static std::enable_if_t< M==3, void > | Serialize (const ParticleSystemData< 3 > &particles, flatbuffers::FlatBufferBuilder *builder, flatbuffers::Offset< fbs::ParticleSystemData3 > *fbsParticleSystemData) |
template<size_t M = N> | |
static std::enable_if_t< M==2, void > | Deserialize (const fbs::ParticleSystemData2 *fbsParticleSystemData, ParticleSystemData< 2 > &particles) |
template<size_t M = N> | |
static std::enable_if_t< M==3, void > | Deserialize (const fbs::ParticleSystemData3 *fbsParticleSystemData, ParticleSystemData< 3 > &particles) |
Detailed Description
template<size_t N>
class CubbyFlow::ParticleSystemData< N >
N-D particle system data.
This class is the key data structure for storing particle system data. A single particle has position, velocity, and force attributes by default. But it can also have additional custom scalar or vector attributes.
Member Typedef Documentation
◆ ScalarData
using CubbyFlow::ParticleSystemData< N >::ScalarData = Array1<double> |
Scalar data chunk.
◆ VectorData
using CubbyFlow::ParticleSystemData< N >::VectorData = Array1<Vector<double, N> > |
Vector data chunk.
Constructor & Destructor Documentation
◆ ParticleSystemData() [1/4]
CubbyFlow::ParticleSystemData< N >::ParticleSystemData | ( | ) |
Default constructor.
◆ ParticleSystemData() [2/4]
|
explicit |
Constructs particle system data with given number of particles.
◆ ~ParticleSystemData()
|
overridedefault |
Default virtual destructor.
◆ ParticleSystemData() [3/4]
CubbyFlow::ParticleSystemData< N >::ParticleSystemData | ( | const ParticleSystemData< N > & | other | ) |
Copy constructor.
◆ ParticleSystemData() [4/4]
|
noexcept |
Move constructor.
Member Function Documentation
◆ AddParticle()
void CubbyFlow::ParticleSystemData< N >::AddParticle | ( | const Vector< double, N > & | newPosition, |
const Vector< double, N > & | newVelocity = Vector< double, N >() , |
||
const Vector< double, N > & | newForce = Vector< double, N >() |
||
) |
Adds a particle to the data structure.
This function will add a single particle to the data structure. For custom data layers, zeros will be assigned for new particles. However, this will invalidate neighbor searcher and neighbor lists. It is users responsibility to call ParticleSystemData::BuildNeighborSearcher and ParticleSystemData::BuildNeighborLists to refresh those data.
- Parameters
-
[in] newPosition The new position. [in] newVelocity The new velocity. [in] newForce The new force.
◆ AddParticles()
void CubbyFlow::ParticleSystemData< N >::AddParticles | ( | const ConstArrayView1< Vector< double, N >> & | newPositions, |
const ConstArrayView1< Vector< double, N >> & | newVelocities = ConstArrayView1< Vector< double, N >>() , |
||
const ConstArrayView1< Vector< double, N >> & | newForces = ConstArrayView1< Vector< double, N >>() |
||
) |
Adds particles to the data structure.
This function will add particles to the data structure. For custom data layers, zeros will be assigned for new particles. However, this will invalidate neighbor searcher and neighbor lists. It is users responsibility to call ParticleSystemData::BuildNeighborSearcher and ParticleSystemData::BuildNeighborLists to refresh those data.
- Parameters
-
[in] newPositions The new positions. [in] newVelocities The new velocities. [in] newForces The new forces.
◆ AddScalarData()
size_t CubbyFlow::ParticleSystemData< N >::AddScalarData | ( | double | initialVal = 0.0 | ) |
Adds a scalar data layer and returns its index.
This function adds a new scalar data layer to the system. It can be used for adding a scalar attribute, such as temperature, to the particles.
- Parameters
-
[in] initialVal Initial value of the new scalar data.
◆ AddVectorData()
size_t CubbyFlow::ParticleSystemData< N >::AddVectorData | ( | const Vector< double, N > & | initialVal = Vector< double, N >{} | ) |
Adds a vector data layer and returns its index.
This function adds a new vector data layer to the system. It can be used for adding a vector attribute, such as vortex, to the particles.
- Parameters
-
[in] initialVal Initial value of the new vector data.
◆ BuildNeighborLists()
void CubbyFlow::ParticleSystemData< N >::BuildNeighborLists | ( | double | maxSearchRadius | ) |
Builds neighbor lists with given search radius.
◆ BuildNeighborSearcher()
void CubbyFlow::ParticleSystemData< N >::BuildNeighborSearcher | ( | double | maxSearchRadius | ) |
Builds neighbor searcher with given search radius.
◆ Deserialize() [1/3]
|
overridevirtual |
Deserializes this particle system data from the buffer.
Implements CubbyFlow::Serializable.
Reimplemented in CubbyFlow::SPHSystemData< N >.
◆ Deserialize() [2/3]
|
staticprotected |
◆ Deserialize() [3/3]
|
staticprotected |
◆ Forces() [1/2]
ConstArrayView1<Vector<double, N> > CubbyFlow::ParticleSystemData< N >::Forces | ( | ) | const |
Returns the force array (immutable).
◆ Forces() [2/2]
ArrayView1<Vector<double, N> > CubbyFlow::ParticleSystemData< N >::Forces | ( | ) |
Returns the force array (mutable).
◆ Mass()
double CubbyFlow::ParticleSystemData< N >::Mass | ( | ) | const |
Returns the mass of the particles.
◆ NeighborLists()
const Array1<Array1<size_t> >& CubbyFlow::ParticleSystemData< N >::NeighborLists | ( | ) | const |
Returns neighbor lists.
This function returns neighbor lists which is available after calling PointParallelHashGridSearcher2::BuildNeighborLists. Each list stores indices of the neighbors.
- Returns
- Neighbor lists.
◆ NeighborSearcher()
const std::shared_ptr<PointNeighborSearcher<N> >& CubbyFlow::ParticleSystemData< N >::NeighborSearcher | ( | ) | const |
Returns neighbor searcher.
This function returns currently set neighbor searcher object. By default, PointParallelHashGridSearcher2 is used.
- Returns
- Current neighbor searcher.
◆ NumberOfParticles()
size_t CubbyFlow::ParticleSystemData< N >::NumberOfParticles | ( | ) | const |
Returns the number of particles.
◆ operator=() [1/2]
ParticleSystemData& CubbyFlow::ParticleSystemData< N >::operator= | ( | const ParticleSystemData< N > & | other | ) |
Copy assignment operator.
◆ operator=() [2/2]
|
noexcept |
Move assignment operator.
◆ Positions() [1/2]
ConstArrayView1<Vector<double, N> > CubbyFlow::ParticleSystemData< N >::Positions | ( | ) | const |
Returns the position array (immutable).
◆ Positions() [2/2]
ArrayView1<Vector<double, N> > CubbyFlow::ParticleSystemData< N >::Positions | ( | ) |
Returns the position array (mutable).
◆ Radius()
double CubbyFlow::ParticleSystemData< N >::Radius | ( | ) | const |
Returns the radius of the particles.
◆ Resize()
void CubbyFlow::ParticleSystemData< N >::Resize | ( | size_t | newNumberOfParticles | ) |
Resizes the number of particles of the container.
This function will resize internal containers to store newly given number of particles including custom data layers. However, this will invalidate neighbor searcher and neighbor lists. It is users responsibility to call ParticleSystemData::BuildNeighborSearcher and ParticleSystemData::BuildNeighborLists to refresh those data.
- Parameters
-
[in] newNumberOfParticles New number of particles.
◆ ScalarDataAt() [1/2]
ConstArrayView1<double> CubbyFlow::ParticleSystemData< N >::ScalarDataAt | ( | size_t | idx | ) | const |
Returns custom scalar data layer at given index (immutable).
◆ ScalarDataAt() [2/2]
ArrayView1<double> CubbyFlow::ParticleSystemData< N >::ScalarDataAt | ( | size_t | idx | ) |
Returns custom scalar data layer at given index (mutable).
◆ Serialize() [1/3]
|
overridevirtual |
Serializes this particle system data to the buffer.
Implements CubbyFlow::Serializable.
Reimplemented in CubbyFlow::SPHSystemData< N >.
◆ Serialize() [2/3]
|
staticprotected |
◆ Serialize() [3/3]
|
staticprotected |
◆ Set()
void CubbyFlow::ParticleSystemData< N >::Set | ( | const ParticleSystemData< N > & | other | ) |
Copies from other particle system data.
◆ SetMass()
|
virtual |
Sets the mass of the particles.
Reimplemented in CubbyFlow::SPHSystemData< N >.
◆ SetNeighborSearcher()
void CubbyFlow::ParticleSystemData< N >::SetNeighborSearcher | ( | const std::shared_ptr< PointNeighborSearcher< N >> & | newNeighborSearcher | ) |
Sets neighbor searcher.
◆ SetRadius()
|
virtual |
Sets the radius of the particles.
Reimplemented in CubbyFlow::SPHSystemData< N >.
◆ VectorDataAt() [1/2]
ConstArrayView1<Vector<double, N> > CubbyFlow::ParticleSystemData< N >::VectorDataAt | ( | size_t | idx | ) | const |
Returns custom vector data layer at given index (immutable).
◆ VectorDataAt() [2/2]
ArrayView1<Vector<double, N> > CubbyFlow::ParticleSystemData< N >::VectorDataAt | ( | size_t | idx | ) |
Returns custom vector data layer at given index (mutable).
◆ Velocities() [1/2]
ConstArrayView1<Vector<double, N> > CubbyFlow::ParticleSystemData< N >::Velocities | ( | ) | const |
Returns the velocity array (immutable).
◆ Velocities() [2/2]
ArrayView1<Vector<double, N> > CubbyFlow::ParticleSystemData< N >::Velocities | ( | ) |
Returns the velocity array (mutable).
The documentation for this class was generated from the following file:
- Core/Particle/ParticleSystemData.hpp