Hash grid-based N-D point searcher. More...
#include <Core/Searcher/PointHashGridSearcher.hpp>
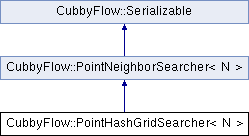
Classes | |
class | Builder |
Front-end to create PointHashGridSearcher objects step by step. More... | |
Public Member Functions | |
PointHashGridSearcher (const Vector< size_t, N > &resolution, double gridSpacing) | |
Constructs hash grid with given resolution and grid spacing. More... | |
~PointHashGridSearcher () override=default | |
Default virtual destructor. More... | |
PointHashGridSearcher (const PointHashGridSearcher &other) | |
Copy constructor. More... | |
PointHashGridSearcher (PointHashGridSearcher &&other) noexcept | |
Move constructor. More... | |
PointHashGridSearcher & | operator= (const PointHashGridSearcher &other) |
Copy assignment operator. More... | |
PointHashGridSearcher & | operator= (PointHashGridSearcher &&other) noexcept |
Move assignment operator. More... | |
void | Build (const ConstArrayView1< Vector< double, N >> &points, double maxSearchRadius) override |
void | ForEachNearbyPoint (const Vector< double, N > &origin, double radius, const ForEachNearbyPointFunc &callback) const override |
bool | HasNearbyPoint (const Vector< double, N > &origin, double radius) const override |
void | Add (const Vector< double, N > &point) |
Adds a single point to the hash grid. More... | |
const Array1< Array1< size_t > > & | Buckets () const |
Returns the internal bucket. More... | |
std::shared_ptr< PointNeighborSearcher< N > > | Clone () const override |
Creates a new instance of the object with same properties than original. More... | |
void | Set (const PointHashGridSearcher &other) |
Copy from the other instance. More... | |
void | Serialize (std::vector< uint8_t > *buffer) const override |
Serializes the neighbor searcher into the buffer. More... | |
void | Deserialize (const std::vector< uint8_t > &buffer) override |
Deserializes the neighbor searcher from the buffer. More... | |
![]() | |
PointNeighborSearcher ()=default | |
Default constructor. More... | |
~PointNeighborSearcher () override=default | |
Default virtual destructor. More... | |
PointNeighborSearcher (const PointNeighborSearcher &other)=default | |
Default copy constructor. More... | |
PointNeighborSearcher (PointNeighborSearcher &&other) noexcept=default | |
Default move constructor. More... | |
PointNeighborSearcher & | operator= (const PointNeighborSearcher &other)=default |
Default copy assignment operator. More... | |
PointNeighborSearcher & | operator= (PointNeighborSearcher &&other) noexcept=default |
Default move assignment operator. More... | |
virtual std::string | TypeName () const =0 |
Returns the type name of the derived class. More... | |
virtual void | Build (const ConstArrayView1< Vector< double, N >> &points) |
Builds internal acceleration structure for given points list. More... | |
![]() | |
Serializable ()=default | |
Default constructor. More... | |
Serializable (const Serializable &)=default | |
Default copy constructor. More... | |
Serializable (Serializable &&) noexcept=default | |
Default move constructor. More... | |
virtual | ~Serializable ()=default |
Default virtual destructor. More... | |
Serializable & | operator= (const Serializable &)=default |
Default copy assignment operator. More... | |
Serializable & | operator= (Serializable &&) noexcept=default |
Default move assignment operator. More... | |
Static Public Member Functions | |
static Builder | GetBuilder () |
Returns builder fox PointHashGridSearcher. More... | |
Additional Inherited Members | |
![]() | |
using | ForEachNearbyPointFunc = std::function< void(size_t, const Vector< double, N > &)> |
Detailed Description
template<size_t N>
class CubbyFlow::PointHashGridSearcher< N >
Hash grid-based N-D point searcher.
This class implements N-D point searcher by using hash grid for its internal acceleration data structure. Each point is recorded to its corresponding bucket where the hashing function is N-D grid mapping.
Constructor & Destructor Documentation
◆ PointHashGridSearcher() [1/3]
CubbyFlow::PointHashGridSearcher< N >::PointHashGridSearcher | ( | const Vector< size_t, N > & | resolution, |
double | gridSpacing | ||
) |
Constructs hash grid with given resolution and grid spacing.
This constructor takes hash grid resolution and its grid spacing as its input parameters. The grid spacing must be 2x or greater than search radius.
- Parameters
-
[in] resolution The resolution. [in] gridSpacing The grid spacing.
◆ ~PointHashGridSearcher()
|
overridedefault |
Default virtual destructor.
◆ PointHashGridSearcher() [2/3]
CubbyFlow::PointHashGridSearcher< N >::PointHashGridSearcher | ( | const PointHashGridSearcher< N > & | other | ) |
Copy constructor.
◆ PointHashGridSearcher() [3/3]
|
noexcept |
Move constructor.
Member Function Documentation
◆ Add()
void CubbyFlow::PointHashGridSearcher< N >::Add | ( | const Vector< double, N > & | point | ) |
Adds a single point to the hash grid.
This function adds a single point to the hash grid for future queries. It can be used for a hash grid that is already built by calling function PointHashGridSearcher::build.
- Parameters
-
[in] point The point to be added.
◆ Buckets()
const Array1<Array1<size_t> >& CubbyFlow::PointHashGridSearcher< N >::Buckets | ( | ) | const |
Returns the internal bucket.
A bucket is a list of point indices that has same hash value. This function returns the (immutable) internal bucket structure.
- Returns
- List of buckets.
◆ Build()
|
overridevirtual |
Builds internal acceleration structure for given points list and max search radius.
Implements CubbyFlow::PointNeighborSearcher< N >.
◆ Clone()
|
overridevirtual |
Creates a new instance of the object with same properties than original.
- Returns
- Copy of this object.
Implements CubbyFlow::PointNeighborSearcher< N >.
◆ Deserialize()
|
overridevirtual |
Deserializes the neighbor searcher from the buffer.
Implements CubbyFlow::Serializable.
◆ ForEachNearbyPoint()
|
overridevirtual |
Invokes the callback function for each nearby point around the origin within given radius.
- Parameters
-
[in] origin The origin position. [in] radius The search radius. [in] callback The callback function.
Implements CubbyFlow::PointNeighborSearcher< N >.
◆ GetBuilder()
|
static |
Returns builder fox PointHashGridSearcher.
◆ HasNearbyPoint()
|
overridevirtual |
Returns true if there are any nearby points for given origin within radius.
- Parameters
-
[in] origin The origin. [in] radius The radius.
- Returns
- True if has nearby point, false otherwise.
Implements CubbyFlow::PointNeighborSearcher< N >.
◆ operator=() [1/2]
PointHashGridSearcher& CubbyFlow::PointHashGridSearcher< N >::operator= | ( | const PointHashGridSearcher< N > & | other | ) |
Copy assignment operator.
◆ operator=() [2/2]
|
noexcept |
Move assignment operator.
◆ Serialize()
|
overridevirtual |
Serializes the neighbor searcher into the buffer.
Implements CubbyFlow::Serializable.
◆ Set()
void CubbyFlow::PointHashGridSearcher< N >::Set | ( | const PointHashGridSearcher< N > & | other | ) |
Copy from the other instance.
The documentation for this class was generated from the following file:
- Core/Searcher/PointHashGridSearcher.hpp