KdTree-based N-D point searcher. More...
#include <Core/Searcher/PointKdTreeSearcher.hpp>
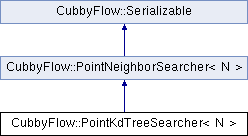
Classes | |
class | Builder |
Front-end to create PointKdTreeSearcher objects step by step. More... | |
Public Member Functions | |
PointKdTreeSearcher ()=default | |
Constructs an empty kD-tree instance. More... | |
~PointKdTreeSearcher () override=default | |
Default virtual destructor. More... | |
PointKdTreeSearcher (const PointKdTreeSearcher &other) | |
Copy constructor. More... | |
PointKdTreeSearcher (PointKdTreeSearcher &&other) noexcept | |
Move constructor. More... | |
PointKdTreeSearcher & | operator= (const PointKdTreeSearcher &other) |
Copy assignment operator. More... | |
PointKdTreeSearcher & | operator= (PointKdTreeSearcher &&other) noexcept |
Move assignment operator. More... | |
void | Build (const ConstArrayView1< Vector< double, N >> &points, double maxSearchRadius) override |
void | ForEachNearbyPoint (const Vector< double, N > &origin, double radius, const ForEachNearbyPointFunc &callback) const override |
bool | HasNearbyPoint (const Vector< double, N > &origin, double radius) const override |
std::shared_ptr< PointNeighborSearcher< N > > | Clone () const override |
Creates a new instance of the object with same properties than original. More... | |
void | Set (const PointKdTreeSearcher &other) |
Copy from the other instance. More... | |
void | Serialize (std::vector< uint8_t > *buffer) const override |
Serializes the neighbor searcher into the buffer. More... | |
void | Deserialize (const std::vector< uint8_t > &buffer) override |
Deserializes the neighbor searcher from the buffer. More... | |
![]() | |
PointNeighborSearcher ()=default | |
Default constructor. More... | |
~PointNeighborSearcher () override=default | |
Default virtual destructor. More... | |
PointNeighborSearcher (const PointNeighborSearcher &other)=default | |
Default copy constructor. More... | |
PointNeighborSearcher (PointNeighborSearcher &&other) noexcept=default | |
Default move constructor. More... | |
PointNeighborSearcher & | operator= (const PointNeighborSearcher &other)=default |
Default copy assignment operator. More... | |
PointNeighborSearcher & | operator= (PointNeighborSearcher &&other) noexcept=default |
Default move assignment operator. More... | |
virtual std::string | TypeName () const =0 |
Returns the type name of the derived class. More... | |
virtual void | Build (const ConstArrayView1< Vector< double, N >> &points) |
Builds internal acceleration structure for given points list. More... | |
![]() | |
Serializable ()=default | |
Default constructor. More... | |
Serializable (const Serializable &)=default | |
Default copy constructor. More... | |
Serializable (Serializable &&) noexcept=default | |
Default move constructor. More... | |
virtual | ~Serializable ()=default |
Default virtual destructor. More... | |
Serializable & | operator= (const Serializable &)=default |
Default copy assignment operator. More... | |
Serializable & | operator= (Serializable &&) noexcept=default |
Default move assignment operator. More... | |
Static Public Member Functions | |
static Builder | GetBuilder () |
Returns builder fox PointKdTreeSearcher. More... | |
Additional Inherited Members | |
![]() | |
using | ForEachNearbyPointFunc = std::function< void(size_t, const Vector< double, N > &)> |
Detailed Description
template<size_t N>
class CubbyFlow::PointKdTreeSearcher< N >
KdTree-based N-D point searcher.
This class implements N-D point searcher by using KdTree for its internal acceleration data structure.
Constructor & Destructor Documentation
◆ PointKdTreeSearcher() [1/3]
|
default |
Constructs an empty kD-tree instance.
◆ ~PointKdTreeSearcher()
|
overridedefault |
Default virtual destructor.
◆ PointKdTreeSearcher() [2/3]
CubbyFlow::PointKdTreeSearcher< N >::PointKdTreeSearcher | ( | const PointKdTreeSearcher< N > & | other | ) |
Copy constructor.
◆ PointKdTreeSearcher() [3/3]
|
noexcept |
Move constructor.
Member Function Documentation
◆ Build()
|
overridevirtual |
Builds internal acceleration structure for given points list and max search radius.
Implements CubbyFlow::PointNeighborSearcher< N >.
◆ Clone()
|
overridevirtual |
Creates a new instance of the object with same properties than original.
- Returns
- Copy of this object.
Implements CubbyFlow::PointNeighborSearcher< N >.
◆ Deserialize()
|
overridevirtual |
Deserializes the neighbor searcher from the buffer.
Implements CubbyFlow::Serializable.
◆ ForEachNearbyPoint()
|
overridevirtual |
Invokes the callback function for each nearby point around the origin within given radius.
- Parameters
-
[in] origin The origin position. [in] radius The search radius. [in] callback The callback function.
Implements CubbyFlow::PointNeighborSearcher< N >.
◆ GetBuilder()
|
static |
Returns builder fox PointKdTreeSearcher.
◆ HasNearbyPoint()
|
overridevirtual |
Returns true if there are any nearby points for given origin within radius.
- Parameters
-
[in] origin The origin. [in] radius The radius.
- Returns
- True if has nearby point, false otherwise.
Implements CubbyFlow::PointNeighborSearcher< N >.
◆ operator=() [1/2]
PointKdTreeSearcher& CubbyFlow::PointKdTreeSearcher< N >::operator= | ( | const PointKdTreeSearcher< N > & | other | ) |
Copy assignment operator.
◆ operator=() [2/2]
|
noexcept |
Move assignment operator.
◆ Serialize()
|
overridevirtual |
Serializes the neighbor searcher into the buffer.
Implements CubbyFlow::Serializable.
◆ Set()
void CubbyFlow::PointKdTreeSearcher< N >::Set | ( | const PointKdTreeSearcher< N > & | other | ) |
Copy from the other instance.
The documentation for this class was generated from the following file:
- Core/Searcher/PointKdTreeSearcher.hpp