Classes |
Public Member Functions |
Static Public Member Functions |
Public Attributes |
Protected Member Functions |
List of all members
CubbyFlow::Cylinder3 Class Referencefinal
3-D cylinder geometry. More...
#include <Core/Geometry/Cylinder3.hpp>
Inheritance diagram for CubbyFlow::Cylinder3:
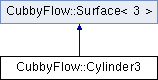
Classes | |
class | Builder |
Front-end to create Cylinder3 objects step by step. More... | |
Public Member Functions | |
Cylinder3 (const Transform3 &_transform=Transform3{}, bool _isNormalFlipped=false) | |
Constructs a cylinder with _transform and _isNormalFlipped . More... | |
Cylinder3 (Vector3D _center, double _radius, double _height, const Transform3 &_transform=Transform3{}, bool _isNormalFlipped=false) | |
Cylinder3 (const Cylinder3 &)=default | |
Default copy constructor. More... | |
Cylinder3 (Cylinder3 &&) noexcept=default | |
Default move constructor. More... | |
~Cylinder3 () override=default | |
Default virtual destructor. More... | |
Cylinder3 & | operator= (const Cylinder3 &)=default |
Default copy assignment operator. More... | |
Cylinder3 & | operator= (Cylinder3 &&) noexcept=default |
Default move assignment operator. More... | |
![]() | |
Surface (const Transform< N > &transform=Transform< N >(), bool isNormalFlipped=false) | |
Constructs a surface with normal direction. More... | |
virtual | ~Surface ()=default |
Default virtual destructor. More... | |
Surface (const Surface &other) | |
Copy constructor. More... | |
Surface (Surface &&other) noexcept | |
Move constructor. More... | |
Surface & | operator= (const Surface &other) |
Copy assignment operator. More... | |
Surface & | operator= (Surface &&other) noexcept |
Move assignment operator. More... | |
Vector< double, N > | ClosestPoint (const Vector< double, N > &otherPoint) const |
BoundingBox< double, N > | GetBoundingBox () const |
Returns the bounding box of this surface object. More... | |
bool | Intersects (const Ray< double, N > &ray) const |
Returns true if the given ray intersects with this surface object. More... | |
double | ClosestDistance (const Vector< double, N > &otherPoint) const |
SurfaceRayIntersection< N > | ClosestIntersection (const Ray< double, N > &ray) const |
Returns the closest intersection point for given ray . More... | |
Vector< double, N > | ClosestNormal (const Vector< double, N > &otherPoint) const |
virtual void | UpdateQueryEngine () |
Updates internal spatial query engine. More... | |
virtual bool | IsBounded () const |
Returns true if bounding box can be defined. More... | |
virtual bool | IsValidGeometry () const |
Returns true if the surface is a valid geometry. More... | |
bool | IsInside (const Vector< double, N > &otherPoint) const |
Static Public Member Functions | |
static Builder | GetBuilder () |
Returns builder fox Cylinder3. More... | |
Public Attributes | |
Vector3D | center |
Center of the cylinder. More... | |
double | radius = 1.0 |
Radius of the cylinder. More... | |
double | height = 1.0 |
Height of the cylinder. More... | |
![]() | |
Transform< N > | transform |
Local-to-world transform. More... | |
bool | isNormalFlipped = false |
Flips normal. More... | |
Protected Member Functions | |
Vector3D | ClosestPointLocal (const Vector3D &otherPoint) const override |
double | ClosestDistanceLocal (const Vector3D &otherPoint) const override |
bool | IntersectsLocal (const Ray3D &ray) const override |
BoundingBox3D | BoundingBoxLocal () const override |
Returns the bounding box of this surface object in local frame. More... | |
Vector3D | ClosestNormalLocal (const Vector3D &otherPoint) const override |
SurfaceRayIntersection3 | ClosestIntersectionLocal (const Ray3D &ray) const override |
![]() | |
virtual Vector< double, N > | ClosestPointLocal (const Vector< double, N > &otherPoint) const =0 |
virtual SurfaceRayIntersection< N > | ClosestIntersectionLocal (const Ray< double, N > &ray) const =0 |
Returns the closest intersection point for given ray in local frame. More... | |
virtual Vector< double, N > | ClosestNormalLocal (const Vector< double, N > &otherPoint) const =0 |
virtual bool | IntersectsLocal (const Ray< double, N > &ray) const |
virtual double | ClosestDistanceLocal (const Vector< double, N > &otherPoint) const |
virtual bool | IsInsideLocal (const Vector< double, N > &otherPoint) const |
Detailed Description
3-D cylinder geometry.
This class represents 3-D cylinder geometry which extends Surface3 by overriding surface-related queries. The cylinder is aligned with the y-axis.
Constructor & Destructor Documentation
◆ Cylinder3() [1/4]
CubbyFlow::Cylinder3::Cylinder3 | ( | const Transform3 & | _transform = Transform3{} , |
bool | _isNormalFlipped = false |
||
) |
Constructs a cylinder with _transform
and _isNormalFlipped
.
◆ Cylinder3() [2/4]
CubbyFlow::Cylinder3::Cylinder3 | ( | Vector3D | _center, |
double | _radius, | ||
double | _height, | ||
const Transform3 & | _transform = Transform3{} , |
||
bool | _isNormalFlipped = false |
||
) |
Constructs a cylinder with _center
, _radius
, _height
, _transform
and _isNormalFlipped
.
◆ Cylinder3() [3/4]
|
default |
Default copy constructor.
◆ Cylinder3() [4/4]
|
defaultnoexcept |
Default move constructor.
◆ ~Cylinder3()
|
overridedefault |
Default virtual destructor.
Member Function Documentation
◆ BoundingBoxLocal()
|
overrideprotectedvirtual |
Returns the bounding box of this surface object in local frame.
Implements CubbyFlow::Surface< N >.
◆ ClosestDistanceLocal()
|
overrideprotected |
◆ ClosestIntersectionLocal()
|
overrideprotected |
◆ ClosestNormalLocal()
|
overrideprotected |
◆ ClosestPointLocal()
|
overrideprotected |
◆ GetBuilder()
◆ IntersectsLocal()
|
overrideprotected |
◆ operator=() [1/2]
Default copy assignment operator.
◆ operator=() [2/2]
Default move assignment operator.
Member Data Documentation
◆ center
Vector3D CubbyFlow::Cylinder3::center |
Center of the cylinder.
◆ height
double CubbyFlow::Cylinder3::height = 1.0 |
Height of the cylinder.
◆ radius
double CubbyFlow::Cylinder3::radius = 1.0 |
Radius of the cylinder.
The documentation for this class was generated from the following file:
- Core/Geometry/Cylinder3.hpp