N-D face-centered (a.k.a MAC or staggered) grid. More...
#include <Core/Grid/FaceCenteredGrid.hpp>
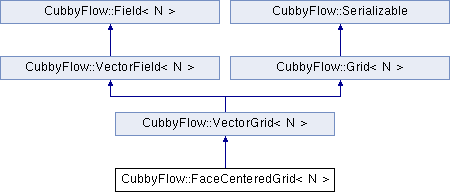
Classes | |
class | Builder |
Front-end to create FaceCenteredGrid objects step by step. More... | |
Public Types | |
using | ScalarDataView = ArrayView< double, N > |
Read-write scalar data view type. More... | |
using | ConstScalarDataView = ArrayView< const double, N > |
Read-only scalar data view type. More... | |
![]() | |
using | VectorDataView = ArrayView< Vector< double, N >, N > |
Read-write array view type. More... | |
using | ConstVectorDataView = ArrayView< const Vector< double, N >, N > |
Read-only array view type. More... | |
Public Member Functions | |
FaceCenteredGrid () | |
Constructs empty grid. More... | |
FaceCenteredGrid (const Vector< size_t, N > &resolution, const Vector< double, N > &gridSpacing=Vector< double, N >::MakeConstant(1.0), const Vector< double, N > &origin=Vector< double, N >{}, const Vector< double, N > &initialValue=Vector< double, N >{}) | |
Resizes the grid using given parameters. More... | |
~FaceCenteredGrid () override=default | |
Default virtual destructor. More... | |
FaceCenteredGrid (const FaceCenteredGrid &other) | |
Copy constructor. More... | |
FaceCenteredGrid (FaceCenteredGrid &&other) noexcept | |
Move constructor. More... | |
FaceCenteredGrid & | operator= (const FaceCenteredGrid &other) |
Copy assignment operator. More... | |
FaceCenteredGrid & | operator= (FaceCenteredGrid &&other) noexcept |
Move assignment operator. More... | |
void | Swap (Grid< N > *other) override |
Swaps the contents with the given other grid. More... | |
void | Set (const FaceCenteredGrid &other) |
Sets the contents with the given other grid. More... | |
double & | U (const Vector< size_t, N > &idx) |
Returns u-value at given data point. More... | |
template<typename... Indices> | |
double & | U (size_t i, Indices... indices) |
Returns u-value at given data point. More... | |
const double & | U (const Vector< size_t, N > &idx) const |
Returns u-value at given data point. More... | |
template<typename... Indices> | |
const double & | U (size_t i, Indices... indices) const |
Returns u-value at given data point. More... | |
double & | V (const Vector< size_t, N > &idx) |
Returns v-value at given data point. More... | |
template<typename... Indices> | |
double & | V (size_t i, Indices... indices) |
Returns v-value at given data point. More... | |
const double & | V (const Vector< size_t, N > &idx) const |
Returns v-value at given data point. More... | |
template<typename... Indices> | |
const double & | V (size_t i, Indices... indices) const |
Returns v-value at given data point. More... | |
template<size_t M = N> | |
std::enable_if_t< M==3, double & > | W (const Vector< size_t, N > &idx) |
Returns w-value at given data point. More... | |
template<size_t M = N, typename... Indices> | |
std::enable_if_t< M==3, double & > | W (size_t i, Indices... indices) |
Returns w-value at given data point. More... | |
template<size_t M = N> | |
std::enable_if_t< M==3, const double & > | W (const Vector< size_t, N > &idx) const |
Returns w-value at given data point. More... | |
template<size_t M = N, typename... Indices> | |
std::enable_if_t< M==3, const double & > | W (size_t i, Indices... indices) const |
Returns w-value at given data point. More... | |
Vector< double, N > | ValueAtCellCenter (const Vector< size_t, N > &idx) const |
Returns interpolated value at cell center. More... | |
template<typename... Indices> | |
Vector< double, N > | ValueAtCellCenter (size_t i, Indices... indices) const |
Returns interpolated value at cell center. More... | |
double | DivergenceAtCellCenter (const Vector< size_t, N > &idx) const |
Returns divergence at cell-center location. More... | |
template<typename... Indices> | |
double | DivergenceAtCellCenter (size_t i, Indices... indices) const |
Returns divergence at cell-center location. More... | |
GetCurl< N >::Type | CurlAtCellCenter (const Vector< size_t, N > &idx) const |
Returns curl at cell-center location. More... | |
template<typename... Indices> | |
GetCurl< N >::Type | CurlAtCellCenter (size_t i, Indices... indices) const |
Returns curl at cell-center location. More... | |
ScalarDataView | UView () |
Returns u data view. More... | |
ConstScalarDataView | UView () const |
Returns read-only u data view. More... | |
ScalarDataView | VView () |
Returns v data view. More... | |
ConstScalarDataView | VView () const |
Returns read-only v data view. More... | |
template<size_t M = N> | |
std::enable_if_t< M==3, ScalarDataView > | WView () |
Returns w data view. More... | |
template<size_t M = N> | |
std::enable_if_t< M==3, ConstScalarDataView > | WView () const |
Returns read-only w data view. More... | |
ScalarDataView | DataView (size_t i) |
Returns i-th data view. More... | |
ConstScalarDataView | DataView (size_t i) const |
Returns read-only i-th data view. More... | |
GridDataPositionFunc< N > | UPosition () const |
Returns function object that maps u data point to its actual position. More... | |
GridDataPositionFunc< N > | VPosition () const |
Returns function object that maps v data point to its actual position. More... | |
template<size_t M = N> | |
std::enable_if_t< M==3, GridDataPositionFunc< N > > | WPosition () const |
Returns function object that maps w data point to its actual position. More... | |
GridDataPositionFunc< N > | DataPosition (size_t i) const |
Returns function object that maps data point to its actual position. More... | |
Vector< size_t, N > | USize () const |
Returns data size of the u component. More... | |
Vector< size_t, N > | VSize () const |
Returns data size of the v component. More... | |
template<size_t M = N> | |
std::enable_if_t< M==3, Vector< size_t, N > > | WSize () const |
Returns data size of the w component. More... | |
Vector< size_t, N > | DataSize (size_t i) const |
Returns data size of the i-th component. More... | |
Vector< double, N > | UOrigin () const |
Returns u-data position for the grid point at (0, 0, ...). More... | |
Vector< double, N > | VOrigin () const |
Returns v-data position for the grid point at (0, 0, ...). More... | |
template<size_t M = N> | |
std::enable_if_t< M==3, Vector< double, N > > | WOrigin () const |
Returns w-data position for the grid point at (0, 0, ...). More... | |
Vector< double, N > | DataOrigin (size_t i) const |
Returns i-th data position for the grid point at (0, 0, ...). More... | |
void | Fill (const Vector< double, N > &value, ExecutionPolicy policy=ExecutionPolicy::Parallel) override |
Fills the grid with given value. More... | |
void | Fill (const std::function< Vector< double, N >(const Vector< double, N > &)> &func, ExecutionPolicy policy=ExecutionPolicy::Parallel) override |
Fills the grid with given function. More... | |
std::shared_ptr< VectorGrid< N > > | Clone () const override |
Returns the copy of the grid instance. More... | |
void | ForEachUIndex (const std::function< void(const Vector< size_t, N > &)> &func) const |
Invokes the given function func for each u-data point. More... | |
void | ParallelForEachUIndex (const std::function< void(const Vector< size_t, N > &)> &func) const |
Invokes the given function func for each u-data point in parallel. More... | |
void | ForEachVIndex (const std::function< void(const Vector< size_t, N > &)> &func) const |
Invokes the given function func for each v-data point. More... | |
void | ParallelForEachVIndex (const std::function< void(const Vector< size_t, N > &)> &func) const |
Invokes the given function func for each v-data point in parallel. More... | |
template<size_t M = N> | |
std::enable_if_t< M==3, void > | ForEachWIndex (const std::function< void(const Vector< size_t, N > &)> &func) const |
Invokes the given function func for each w-data point. More... | |
template<size_t M = N> | |
std::enable_if_t< M==3, void > | ParallelForEachWIndex (const std::function< void(const Vector< size_t, N > &)> &func) const |
Invokes the given function func for each w-data point parallelly. More... | |
Vector< double, N > | Sample (const Vector< double, N > &x) const override |
Returns sampled value at given position x . More... | |
double | Divergence (const Vector< double, N > &x) const override |
Returns divergence at given position x . More... | |
GetCurl< N >::Type | Curl (const Vector< double, N > &x) const override |
Returns curl at given position x . More... | |
std::function< Vector< double, N >const Vector< double, N > &)> | Sampler () const override |
Returns the sampler function. More... | |
![]() | |
VectorGrid ()=default | |
Constructs an empty grid. More... | |
~VectorGrid () override=default | |
Default virtual destructor. More... | |
VectorGrid (const VectorGrid &other) | |
Copy constructor. More... | |
VectorGrid (VectorGrid &&other) noexcept | |
Move constructor. More... | |
VectorGrid & | operator= (const VectorGrid &other) |
Copy assignment operator. More... | |
VectorGrid & | operator= (VectorGrid &&other) noexcept |
Move assignment operator. More... | |
void | Clear () |
Clears the contents of the grid. More... | |
void | Resize (const Vector< size_t, N > &resolution, const Vector< double, N > &gridSpacing=Vector< double, N >::MakeConstant(1.0), const Vector< double, N > &origin=Vector< double, N >{}, const Vector< double, N > &initialValue=Vector< double, N >{}) |
Resizes the grid using given parameters. More... | |
void | Resize (const Vector< double, N > &gridSpacing, const Vector< double, N > &origin) |
Resizes the grid using given parameters. More... | |
void | Serialize (std::vector< uint8_t > *buffer) const override |
Serializes the grid instance to the output buffer. More... | |
void | Deserialize (const std::vector< uint8_t > &buffer) override |
Deserializes the input buffer to the grid instance. More... | |
![]() | |
VectorField ()=default | |
Default constructor. More... | |
~VectorField () override=default | |
Default destructor. More... | |
VectorField (const VectorField &)=default | |
Default copy constructor. More... | |
VectorField (VectorField &&) noexcept=default | |
Default move constructor. More... | |
VectorField & | operator= (const VectorField &)=default |
Default copy assignment operator. More... | |
VectorField & | operator= (VectorField &&) noexcept=default |
Default move assignment operator. More... | |
![]() | |
Field ()=default | |
Default constructor. More... | |
virtual | ~Field ()=default |
Default virtual destructor. More... | |
Field (const Field &)=default | |
Default copy constructor. More... | |
Field (Field &&) noexcept=default | |
Default move constructor. More... | |
Field & | operator= (const Field &)=default |
Default copy assignment operator. More... | |
Field & | operator= (Field &&) noexcept=default |
Default move assignment operator. More... | |
![]() | |
Grid ()=default | |
Constructs an empty grid. More... | |
~Grid () override=default | |
Default virtual destructor. More... | |
Grid (const Grid &other) | |
Copy constructor. More... | |
Grid (Grid &&other) noexcept | |
Move constructor. More... | |
Grid & | operator= (const Grid &other) |
Copy assignment operator. More... | |
Grid & | operator= (Grid &&other) noexcept |
Move assignment operator. More... | |
virtual std::string | TypeName () const =0 |
Returns the type name of derived grid. More... | |
const Vector< size_t, N > & | Resolution () const |
Returns the grid resolution. More... | |
const Vector< double, N > & | Origin () const |
Returns the grid origin. More... | |
const Vector< double, N > & | GridSpacing () const |
Returns the grid spacing. More... | |
const BoundingBox< double, N > & | GetBoundingBox () const |
Returns the bounding box of the grid. More... | |
GridDataPositionFunc< N > | CellCenterPosition () const |
Returns the function that maps grid index to the cell-center position. More... | |
void | ForEachCellIndex (const std::function< void(const Vector< size_t, N > &)> &func) const |
Invokes the given function func for each grid cell. More... | |
template<size_t M = N> | |
std::enable_if_t< M==2, void > | ForEachCellIndex (const std::function< void(size_t, size_t)> &func) const |
template<size_t M = N> | |
std::enable_if_t< M==3, void > | ForEachCellIndex (const std::function< void(size_t, size_t, size_t)> &func) const |
void | ParallelForEachCellIndex (const std::function< void(const Vector< size_t, N > &)> &func) const |
Invokes the given function func for each grid cell in parallel. More... | |
template<size_t M = N> | |
std::enable_if_t< M==2, void > | ParallelForEachCellIndex (const std::function< void(size_t, size_t)> &func) const |
template<size_t M = N> | |
std::enable_if_t< M==3, void > | ParallelForEachCellIndex (const std::function< void(size_t, size_t, size_t)> &func) const |
bool | HasSameShape (const Grid &other) const |
Returns true if resolution, grid-spacing and origin are same. More... | |
![]() | |
Serializable ()=default | |
Default constructor. More... | |
Serializable (const Serializable &)=default | |
Default copy constructor. More... | |
Serializable (Serializable &&) noexcept=default | |
Default move constructor. More... | |
virtual | ~Serializable ()=default |
Default virtual destructor. More... | |
Serializable & | operator= (const Serializable &)=default |
Default copy assignment operator. More... | |
Serializable & | operator= (Serializable &&) noexcept=default |
Default move assignment operator. More... | |
Static Public Member Functions | |
static Builder | GetBuilder () |
Returns builder fox FaceCenteredGrid. More... | |
Protected Member Functions | |
void | OnResize (const Vector< size_t, N > &resolution, const Vector< double, N > &gridSpacing, const Vector< double, N > &origin, const Vector< double, N > &initialValue) final |
Invoked when the resizing happens. More... | |
void | GetData (Array1< double > &data) const override |
Fetches the data into a continuous linear array. More... | |
void | SetData (const ConstArrayView1< double > &data) override |
Sets the data from a continuous linear array. More... | |
![]() | |
void | SetSizeParameters (const Vector< size_t, N > &resolution, const Vector< double, N > &gridSpacing, const Vector< double, N > &origin) |
void | SwapGrid (Grid *other) |
Swaps the size parameters with given grid other . More... | |
void | SetGrid (const Grid &other) |
Sets the size parameters with given grid other . More... | |
Detailed Description
template<size_t N>
class CubbyFlow::FaceCenteredGrid< N >
N-D face-centered (a.k.a MAC or staggered) grid.
This class implements face-centered grid which is also known as marker-and-cell (MAC) or staggered grid. This vector grid stores each vector component at face center. Thus, u, v (and w for 3-D) components are not collocated.
Member Typedef Documentation
◆ ConstScalarDataView
using CubbyFlow::FaceCenteredGrid< N >::ConstScalarDataView = ArrayView<const double, N> |
Read-only scalar data view type.
◆ ScalarDataView
using CubbyFlow::FaceCenteredGrid< N >::ScalarDataView = ArrayView<double, N> |
Read-write scalar data view type.
Constructor & Destructor Documentation
◆ FaceCenteredGrid() [1/4]
CubbyFlow::FaceCenteredGrid< N >::FaceCenteredGrid | ( | ) |
Constructs empty grid.
◆ FaceCenteredGrid() [2/4]
CubbyFlow::FaceCenteredGrid< N >::FaceCenteredGrid | ( | const Vector< size_t, N > & | resolution, |
const Vector< double, N > & | gridSpacing = Vector< double, N >::MakeConstant(1.0) , |
||
const Vector< double, N > & | origin = Vector< double, N >{} , |
||
const Vector< double, N > & | initialValue = Vector< double, N >{} |
||
) |
Resizes the grid using given parameters.
◆ ~FaceCenteredGrid()
|
overridedefault |
Default virtual destructor.
◆ FaceCenteredGrid() [3/4]
CubbyFlow::FaceCenteredGrid< N >::FaceCenteredGrid | ( | const FaceCenteredGrid< N > & | other | ) |
Copy constructor.
◆ FaceCenteredGrid() [4/4]
|
noexcept |
Move constructor.
Member Function Documentation
◆ Clone()
|
overridevirtual |
Returns the copy of the grid instance.
Implements CubbyFlow::VectorGrid< N >.
◆ Curl()
|
overridevirtual |
Returns curl at given position x
.
Reimplemented from CubbyFlow::VectorField< N >.
◆ CurlAtCellCenter() [1/2]
GetCurl<N>::Type CubbyFlow::FaceCenteredGrid< N >::CurlAtCellCenter | ( | const Vector< size_t, N > & | idx | ) | const |
Returns curl at cell-center location.
◆ CurlAtCellCenter() [2/2]
|
inline |
Returns curl at cell-center location.
◆ DataOrigin()
Vector<double, N> CubbyFlow::FaceCenteredGrid< N >::DataOrigin | ( | size_t | i | ) | const |
Returns i-th data position for the grid point at (0, 0, ...).
Note that this is different from origin() since origin() returns the lower corner point of the bounding box.
◆ DataPosition()
GridDataPositionFunc<N> CubbyFlow::FaceCenteredGrid< N >::DataPosition | ( | size_t | i | ) | const |
Returns function object that maps data point to its actual position.
◆ DataSize()
Vector<size_t, N> CubbyFlow::FaceCenteredGrid< N >::DataSize | ( | size_t | i | ) | const |
Returns data size of the i-th component.
◆ DataView() [1/2]
ScalarDataView CubbyFlow::FaceCenteredGrid< N >::DataView | ( | size_t | i | ) |
Returns i-th data view.
◆ DataView() [2/2]
ConstScalarDataView CubbyFlow::FaceCenteredGrid< N >::DataView | ( | size_t | i | ) | const |
Returns read-only i-th data view.
◆ Divergence()
|
overridevirtual |
Returns divergence at given position x
.
Reimplemented from CubbyFlow::VectorField< N >.
◆ DivergenceAtCellCenter() [1/2]
double CubbyFlow::FaceCenteredGrid< N >::DivergenceAtCellCenter | ( | const Vector< size_t, N > & | idx | ) | const |
Returns divergence at cell-center location.
◆ DivergenceAtCellCenter() [2/2]
|
inline |
Returns divergence at cell-center location.
◆ Fill() [1/2]
|
overridevirtual |
Fills the grid with given value.
Implements CubbyFlow::VectorGrid< N >.
◆ Fill() [2/2]
|
overridevirtual |
Fills the grid with given function.
Implements CubbyFlow::VectorGrid< N >.
◆ ForEachUIndex()
void CubbyFlow::FaceCenteredGrid< N >::ForEachUIndex | ( | const std::function< void(const Vector< size_t, N > &)> & | func | ) | const |
Invokes the given function func
for each u-data point.
This function invokes the given function object func
for each u-data point in serial manner. The input parameters are i, j (and k for 3-D) indices of a u-data point. The order of execution is i-first, j-next.
◆ ForEachVIndex()
void CubbyFlow::FaceCenteredGrid< N >::ForEachVIndex | ( | const std::function< void(const Vector< size_t, N > &)> & | func | ) | const |
Invokes the given function func
for each v-data point.
This function invokes the given function object func
for each v-data point in serial manner. The input parameters are i, j (and k for 3-D) indices of a v-data point. The order of execution is i-first, j-next.
◆ ForEachWIndex()
|
inline |
Invokes the given function func
for each w-data point.
This function invokes the given function object func
for each w-data point in serial manner. The input parameters are i, j (and k for 3-D) indices of a w-data point. The order of execution is i-first, j-next.
◆ GetBuilder()
|
static |
Returns builder fox FaceCenteredGrid.
◆ GetData()
|
overrideprotectedvirtual |
Fetches the data into a continuous linear array.
Implements CubbyFlow::Grid< N >.
◆ OnResize()
|
finalprotectedvirtual |
Invoked when the resizing happens.
This callback function is called when the grid gets resized. The overriding class should allocate the internal storage based on its data layout scheme.
Implements CubbyFlow::VectorGrid< N >.
◆ operator=() [1/2]
FaceCenteredGrid& CubbyFlow::FaceCenteredGrid< N >::operator= | ( | const FaceCenteredGrid< N > & | other | ) |
Copy assignment operator.
◆ operator=() [2/2]
|
noexcept |
Move assignment operator.
◆ ParallelForEachUIndex()
void CubbyFlow::FaceCenteredGrid< N >::ParallelForEachUIndex | ( | const std::function< void(const Vector< size_t, N > &)> & | func | ) | const |
Invokes the given function func
for each u-data point in parallel.
This function invokes the given function object func
for each u-data point in parallel manner. The input parameters are i, j (and k for 3-D) indices of a u-data point. The order of execution can be arbitrary since it's multi-threaded.
◆ ParallelForEachVIndex()
void CubbyFlow::FaceCenteredGrid< N >::ParallelForEachVIndex | ( | const std::function< void(const Vector< size_t, N > &)> & | func | ) | const |
Invokes the given function func
for each v-data point in parallel.
This function invokes the given function object func
for each v-data point in parallel manner. The input parameters are i, j (and k for 3-D) indices of a v-data point. The order of execution can be arbitrary since it's multi-threaded.
◆ ParallelForEachWIndex()
|
inline |
Invokes the given function func
for each w-data point parallelly.
This function invokes the given function object func
for each w-data point in parallel manner. The input parameters are i, j (and k for 3-D) indices of a w-data point. The order of execution can be arbitrary since it's multi-threaded.
◆ Sample()
|
overridevirtual |
Returns sampled value at given position x
.
Implements CubbyFlow::VectorField< N >.
◆ Sampler()
|
overridevirtual |
Returns the sampler function.
This function returns the data sampler function object. The sampling function is linear.
Reimplemented from CubbyFlow::VectorField< N >.
◆ Set()
void CubbyFlow::FaceCenteredGrid< N >::Set | ( | const FaceCenteredGrid< N > & | other | ) |
Sets the contents with the given other
grid.
◆ SetData()
|
overrideprotectedvirtual |
Sets the data from a continuous linear array.
Implements CubbyFlow::Grid< N >.
◆ Swap()
|
overridevirtual |
Swaps the contents with the given other
grid.
This function swaps the contents of the grid instance with the given grid object other
only if other
has the same type with this grid.
Implements CubbyFlow::Grid< N >.
◆ U() [1/4]
double& CubbyFlow::FaceCenteredGrid< N >::U | ( | const Vector< size_t, N > & | idx | ) |
Returns u-value at given data point.
◆ U() [2/4]
|
inline |
Returns u-value at given data point.
◆ U() [3/4]
const double& CubbyFlow::FaceCenteredGrid< N >::U | ( | const Vector< size_t, N > & | idx | ) | const |
Returns u-value at given data point.
◆ U() [4/4]
|
inline |
Returns u-value at given data point.
◆ UOrigin()
Vector<double, N> CubbyFlow::FaceCenteredGrid< N >::UOrigin | ( | ) | const |
Returns u-data position for the grid point at (0, 0, ...).
Note that this is different from origin() since origin() returns the lower corner point of the bounding box.
◆ UPosition()
GridDataPositionFunc<N> CubbyFlow::FaceCenteredGrid< N >::UPosition | ( | ) | const |
Returns function object that maps u data point to its actual position.
◆ USize()
Vector<size_t, N> CubbyFlow::FaceCenteredGrid< N >::USize | ( | ) | const |
Returns data size of the u component.
◆ UView() [1/2]
ScalarDataView CubbyFlow::FaceCenteredGrid< N >::UView | ( | ) |
Returns u data view.
◆ UView() [2/2]
ConstScalarDataView CubbyFlow::FaceCenteredGrid< N >::UView | ( | ) | const |
Returns read-only u data view.
◆ V() [1/4]
double& CubbyFlow::FaceCenteredGrid< N >::V | ( | const Vector< size_t, N > & | idx | ) |
Returns v-value at given data point.
◆ V() [2/4]
|
inline |
Returns v-value at given data point.
◆ V() [3/4]
const double& CubbyFlow::FaceCenteredGrid< N >::V | ( | const Vector< size_t, N > & | idx | ) | const |
Returns v-value at given data point.
◆ V() [4/4]
|
inline |
Returns v-value at given data point.
◆ ValueAtCellCenter() [1/2]
Vector<double, N> CubbyFlow::FaceCenteredGrid< N >::ValueAtCellCenter | ( | const Vector< size_t, N > & | idx | ) | const |
Returns interpolated value at cell center.
◆ ValueAtCellCenter() [2/2]
|
inline |
Returns interpolated value at cell center.
◆ VOrigin()
Vector<double, N> CubbyFlow::FaceCenteredGrid< N >::VOrigin | ( | ) | const |
Returns v-data position for the grid point at (0, 0, ...).
Note that this is different from origin() since origin() returns the lower corner point of the bounding box.
◆ VPosition()
GridDataPositionFunc<N> CubbyFlow::FaceCenteredGrid< N >::VPosition | ( | ) | const |
Returns function object that maps v data point to its actual position.
◆ VSize()
Vector<size_t, N> CubbyFlow::FaceCenteredGrid< N >::VSize | ( | ) | const |
Returns data size of the v component.
◆ VView() [1/2]
ScalarDataView CubbyFlow::FaceCenteredGrid< N >::VView | ( | ) |
Returns v data view.
◆ VView() [2/2]
ConstScalarDataView CubbyFlow::FaceCenteredGrid< N >::VView | ( | ) | const |
Returns read-only v data view.
◆ W() [1/4]
|
inline |
Returns w-value at given data point.
◆ W() [2/4]
|
inline |
Returns w-value at given data point.
◆ W() [3/4]
|
inline |
Returns w-value at given data point.
◆ W() [4/4]
|
inline |
Returns w-value at given data point.
◆ WOrigin()
|
inline |
Returns w-data position for the grid point at (0, 0, ...).
Note that this is different from origin() since origin() returns the lower corner point of the bounding box.
◆ WPosition()
|
inline |
Returns function object that maps w data point to its actual position.
◆ WSize()
|
inline |
Returns data size of the w component.
◆ WView() [1/2]
|
inline |
Returns w data view.
◆ WView() [2/2]
|
inline |
Returns read-only w data view.
The documentation for this class was generated from the following file:
- Core/Grid/FaceCenteredGrid.hpp