Abstract base class for N-D cartesian grid structure. More...
#include <Core/Grid/Grid.hpp>
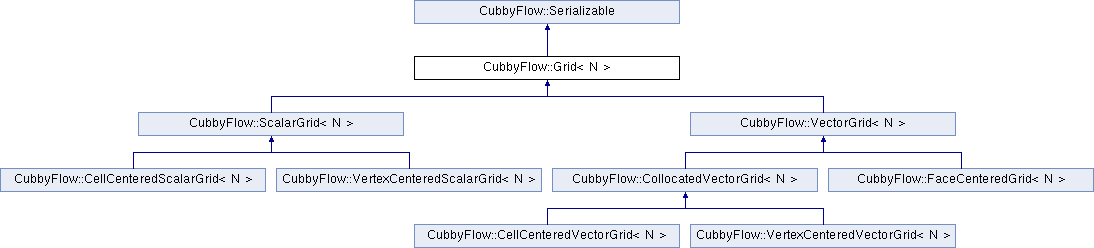
Public Member Functions | |
Grid ()=default | |
Constructs an empty grid. More... | |
~Grid () override=default | |
Default virtual destructor. More... | |
Grid (const Grid &other) | |
Copy constructor. More... | |
Grid (Grid &&other) noexcept | |
Move constructor. More... | |
Grid & | operator= (const Grid &other) |
Copy assignment operator. More... | |
Grid & | operator= (Grid &&other) noexcept |
Move assignment operator. More... | |
virtual std::string | TypeName () const =0 |
Returns the type name of derived grid. More... | |
const Vector< size_t, N > & | Resolution () const |
Returns the grid resolution. More... | |
const Vector< double, N > & | Origin () const |
Returns the grid origin. More... | |
const Vector< double, N > & | GridSpacing () const |
Returns the grid spacing. More... | |
const BoundingBox< double, N > & | GetBoundingBox () const |
Returns the bounding box of the grid. More... | |
GridDataPositionFunc< N > | CellCenterPosition () const |
Returns the function that maps grid index to the cell-center position. More... | |
void | ForEachCellIndex (const std::function< void(const Vector< size_t, N > &)> &func) const |
Invokes the given function func for each grid cell. More... | |
template<size_t M = N> | |
std::enable_if_t< M==2, void > | ForEachCellIndex (const std::function< void(size_t, size_t)> &func) const |
template<size_t M = N> | |
std::enable_if_t< M==3, void > | ForEachCellIndex (const std::function< void(size_t, size_t, size_t)> &func) const |
void | ParallelForEachCellIndex (const std::function< void(const Vector< size_t, N > &)> &func) const |
Invokes the given function func for each grid cell in parallel. More... | |
template<size_t M = N> | |
std::enable_if_t< M==2, void > | ParallelForEachCellIndex (const std::function< void(size_t, size_t)> &func) const |
template<size_t M = N> | |
std::enable_if_t< M==3, void > | ParallelForEachCellIndex (const std::function< void(size_t, size_t, size_t)> &func) const |
bool | HasSameShape (const Grid &other) const |
Returns true if resolution, grid-spacing and origin are same. More... | |
virtual void | Swap (Grid *other)=0 |
Swaps the data with other grid. More... | |
![]() | |
Serializable ()=default | |
Default constructor. More... | |
Serializable (const Serializable &)=default | |
Default copy constructor. More... | |
Serializable (Serializable &&) noexcept=default | |
Default move constructor. More... | |
virtual | ~Serializable ()=default |
Default virtual destructor. More... | |
Serializable & | operator= (const Serializable &)=default |
Default copy assignment operator. More... | |
Serializable & | operator= (Serializable &&) noexcept=default |
Default move assignment operator. More... | |
virtual void | Serialize (std::vector< uint8_t > *buffer) const =0 |
Serializes this instance into the flat buffer. More... | |
virtual void | Deserialize (const std::vector< uint8_t > &buffer)=0 |
Deserializes this instance from the flat buffer. More... | |
Protected Member Functions | |
void | SetSizeParameters (const Vector< size_t, N > &resolution, const Vector< double, N > &gridSpacing, const Vector< double, N > &origin) |
void | SwapGrid (Grid *other) |
Swaps the size parameters with given grid other . More... | |
void | SetGrid (const Grid &other) |
Sets the size parameters with given grid other . More... | |
virtual void | GetData (Array1< double > &data) const =0 |
Fetches the data into a continuous linear array. More... | |
virtual void | SetData (const ConstArrayView1< double > &data)=0 |
Sets the data from a continuous linear array. More... | |
Detailed Description
template<size_t N>
class CubbyFlow::Grid< N >
Abstract base class for N-D cartesian grid structure.
This class represents N-D cartesian grid structure. This class is an abstract base class and does not store any data. The class only stores the shape of the grid. The grid structure is axis-aligned and can have different grid spacing per axis.
Constructor & Destructor Documentation
◆ Grid() [1/3]
|
default |
Constructs an empty grid.
◆ ~Grid()
|
overridedefault |
Default virtual destructor.
◆ Grid() [2/3]
CubbyFlow::Grid< N >::Grid | ( | const Grid< N > & | other | ) |
Copy constructor.
◆ Grid() [3/3]
|
noexcept |
Move constructor.
Member Function Documentation
◆ CellCenterPosition()
GridDataPositionFunc<N> CubbyFlow::Grid< N >::CellCenterPosition | ( | ) | const |
Returns the function that maps grid index to the cell-center position.
◆ ForEachCellIndex() [1/3]
void CubbyFlow::Grid< N >::ForEachCellIndex | ( | const std::function< void(const Vector< size_t, N > &)> & | func | ) | const |
Invokes the given function func
for each grid cell.
This function invokes the given function object func
for each grid cell in serial manner. The input parameters are i and j indices of a grid cell. The order of execution is i-first, j-last.
◆ ForEachCellIndex() [2/3]
|
inline |
◆ ForEachCellIndex() [3/3]
|
inline |
◆ GetBoundingBox()
const BoundingBox<double, N>& CubbyFlow::Grid< N >::GetBoundingBox | ( | ) | const |
Returns the bounding box of the grid.
◆ GetData()
|
protectedpure virtual |
Fetches the data into a continuous linear array.
Implemented in CubbyFlow::FaceCenteredGrid< N >, CubbyFlow::ScalarGrid< N >, and CubbyFlow::CollocatedVectorGrid< N >.
◆ GridSpacing()
const Vector<double, N>& CubbyFlow::Grid< N >::GridSpacing | ( | ) | const |
Returns the grid spacing.
◆ HasSameShape()
bool CubbyFlow::Grid< N >::HasSameShape | ( | const Grid< N > & | other | ) | const |
Returns true if resolution, grid-spacing and origin are same.
◆ operator=() [1/2]
Grid& CubbyFlow::Grid< N >::operator= | ( | const Grid< N > & | other | ) |
Copy assignment operator.
◆ operator=() [2/2]
|
noexcept |
Move assignment operator.
◆ Origin()
const Vector<double, N>& CubbyFlow::Grid< N >::Origin | ( | ) | const |
Returns the grid origin.
◆ ParallelForEachCellIndex() [1/3]
void CubbyFlow::Grid< N >::ParallelForEachCellIndex | ( | const std::function< void(const Vector< size_t, N > &)> & | func | ) | const |
Invokes the given function func
for each grid cell in parallel.
This function invokes the given function object func
for each grid cell in parallel manner. The input parameters are i and j indices of a grid cell. The order of execution can be arbitrary since it's multi-threaded.
◆ ParallelForEachCellIndex() [2/3]
|
inline |
◆ ParallelForEachCellIndex() [3/3]
|
inline |
◆ Resolution()
const Vector<size_t, N>& CubbyFlow::Grid< N >::Resolution | ( | ) | const |
Returns the grid resolution.
◆ SetData()
|
protectedpure virtual |
Sets the data from a continuous linear array.
Implemented in CubbyFlow::FaceCenteredGrid< N >, CubbyFlow::ScalarGrid< N >, and CubbyFlow::CollocatedVectorGrid< N >.
◆ SetGrid()
|
protected |
Sets the size parameters with given grid other
.
◆ SetSizeParameters()
|
protected |
Sets the size parameters including the resolution, grid spacing, and origin.
◆ Swap()
|
pure virtual |
Swaps the data with other grid.
Implemented in CubbyFlow::VertexCenteredScalarGrid< N >, CubbyFlow::VertexCenteredVectorGrid< N >, CubbyFlow::CellCenteredVectorGrid< N >, CubbyFlow::CellCenteredScalarGrid< N >, and CubbyFlow::FaceCenteredGrid< N >.
◆ SwapGrid()
|
protected |
Swaps the size parameters with given grid other
.
◆ TypeName()
|
pure virtual |
Returns the type name of derived grid.
The documentation for this class was generated from the following file:
- Core/Grid/Grid.hpp