3-D triangle geometry. More...
#include <Core/Geometry/Triangle3.hpp>
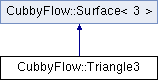
Classes | |
class | Builder |
Front-end to create Triangle3 objects step by step. More... | |
Public Member Functions | |
Triangle3 (const Transform3 &_transform=Transform3{}, bool _isNormalFlipped=false) | |
Constructs an empty triangle. More... | |
Triangle3 (std::array< Vector3D, 3 > points, std::array< Vector3D, 3 > normals, std::array< Vector2D, 3 > uvs, const Transform3 &_transform=Transform3{}, bool _isNormalFlipped=false) | |
Constructs a triangle with given points , normals , and uvs . More... | |
Triangle3 (const Triangle3 &)=default | |
Default copy constructor. More... | |
Triangle3 (Triangle3 &&) noexcept=default | |
Default move constructor. More... | |
~Triangle3 () override=default | |
Default virtual destructor. More... | |
Triangle3 & | operator= (const Triangle3 &)=default |
Default copy assignment operator. More... | |
Triangle3 & | operator= (Triangle3 &&) noexcept=default |
Default move assignment operator. More... | |
double | Area () const |
Returns the area of this triangle. More... | |
void | GetBarycentricCoords (const Vector3D &pt, double *b0, double *b1, double *b2) const |
Returns barycentric coordinates for the given point pt . More... | |
Vector3D | FaceNormal () const |
Returns the face normal of the triangle. More... | |
void | SetNormalsToFaceNormal () |
Set Triangle3::normals to the face normal. More... | |
![]() | |
Surface (const Transform< N > &transform=Transform< N >(), bool isNormalFlipped=false) | |
Constructs a surface with normal direction. More... | |
virtual | ~Surface ()=default |
Default virtual destructor. More... | |
Surface (const Surface &other) | |
Copy constructor. More... | |
Surface (Surface &&other) noexcept | |
Move constructor. More... | |
Surface & | operator= (const Surface &other) |
Copy assignment operator. More... | |
Surface & | operator= (Surface &&other) noexcept |
Move assignment operator. More... | |
Vector< double, N > | ClosestPoint (const Vector< double, N > &otherPoint) const |
BoundingBox< double, N > | GetBoundingBox () const |
Returns the bounding box of this surface object. More... | |
bool | Intersects (const Ray< double, N > &ray) const |
Returns true if the given ray intersects with this surface object. More... | |
double | ClosestDistance (const Vector< double, N > &otherPoint) const |
SurfaceRayIntersection< N > | ClosestIntersection (const Ray< double, N > &ray) const |
Returns the closest intersection point for given ray . More... | |
Vector< double, N > | ClosestNormal (const Vector< double, N > &otherPoint) const |
virtual void | UpdateQueryEngine () |
Updates internal spatial query engine. More... | |
virtual bool | IsBounded () const |
Returns true if bounding box can be defined. More... | |
virtual bool | IsValidGeometry () const |
Returns true if the surface is a valid geometry. More... | |
bool | IsInside (const Vector< double, N > &otherPoint) const |
Static Public Member Functions | |
static Builder | GetBuilder () |
Returns builder fox Triangle3. More... | |
Public Attributes | |
std::array< Vector3D, 3 > | points |
Three points. More... | |
std::array< Vector3D, 3 > | normals |
Three normals. More... | |
std::array< Vector2D, 3 > | uvs |
Three UV coordinates. More... | |
![]() | |
Transform< N > | transform |
Local-to-world transform. More... | |
bool | isNormalFlipped = false |
Flips normal. More... | |
Protected Member Functions | |
Vector3D | ClosestPointLocal (const Vector3D &otherPoint) const override |
bool | IntersectsLocal (const Ray3D &ray) const override |
BoundingBox3D | BoundingBoxLocal () const override |
Returns the bounding box of this surface object in local frame. More... | |
Vector3D | ClosestNormalLocal (const Vector3D &otherPoint) const override |
SurfaceRayIntersection3 | ClosestIntersectionLocal (const Ray3D &ray) const override |
![]() | |
virtual Vector< double, N > | ClosestPointLocal (const Vector< double, N > &otherPoint) const =0 |
virtual SurfaceRayIntersection< N > | ClosestIntersectionLocal (const Ray< double, N > &ray) const =0 |
Returns the closest intersection point for given ray in local frame. More... | |
virtual Vector< double, N > | ClosestNormalLocal (const Vector< double, N > &otherPoint) const =0 |
virtual bool | IntersectsLocal (const Ray< double, N > &ray) const |
virtual double | ClosestDistanceLocal (const Vector< double, N > &otherPoint) const |
virtual bool | IsInsideLocal (const Vector< double, N > &otherPoint) const |
Detailed Description
3-D triangle geometry.
This class represents 3-D triangle geometry which extends Surface3 by overriding surface-related queries.
Constructor & Destructor Documentation
◆ Triangle3() [1/4]
CubbyFlow::Triangle3::Triangle3 | ( | const Transform3 & | _transform = Transform3{} , |
bool | _isNormalFlipped = false |
||
) |
Constructs an empty triangle.
◆ Triangle3() [2/4]
CubbyFlow::Triangle3::Triangle3 | ( | std::array< Vector3D, 3 > | points, |
std::array< Vector3D, 3 > | normals, | ||
std::array< Vector2D, 3 > | uvs, | ||
const Transform3 & | _transform = Transform3{} , |
||
bool | _isNormalFlipped = false |
||
) |
Constructs a triangle with given points
, normals
, and uvs
.
◆ Triangle3() [3/4]
|
default |
Default copy constructor.
◆ Triangle3() [4/4]
|
defaultnoexcept |
Default move constructor.
◆ ~Triangle3()
|
overridedefault |
Default virtual destructor.
Member Function Documentation
◆ Area()
double CubbyFlow::Triangle3::Area | ( | ) | const |
Returns the area of this triangle.
◆ BoundingBoxLocal()
|
overrideprotectedvirtual |
Returns the bounding box of this surface object in local frame.
Implements CubbyFlow::Surface< N >.
◆ ClosestIntersectionLocal()
|
overrideprotected |
◆ ClosestNormalLocal()
|
overrideprotected |
◆ ClosestPointLocal()
|
overrideprotected |
◆ FaceNormal()
Vector3D CubbyFlow::Triangle3::FaceNormal | ( | ) | const |
Returns the face normal of the triangle.
◆ GetBarycentricCoords()
void CubbyFlow::Triangle3::GetBarycentricCoords | ( | const Vector3D & | pt, |
double * | b0, | ||
double * | b1, | ||
double * | b2 | ||
) | const |
Returns barycentric coordinates for the given point pt
.
◆ GetBuilder()
◆ IntersectsLocal()
|
overrideprotected |
◆ operator=() [1/2]
Default copy assignment operator.
◆ operator=() [2/2]
Default move assignment operator.
◆ SetNormalsToFaceNormal()
void CubbyFlow::Triangle3::SetNormalsToFaceNormal | ( | ) |
Set Triangle3::normals to the face normal.
Member Data Documentation
◆ normals
std::array<Vector3D, 3> CubbyFlow::Triangle3::normals |
Three normals.
◆ points
std::array<Vector3D, 3> CubbyFlow::Triangle3::points |
Three points.
◆ uvs
std::array<Vector2D, 3> CubbyFlow::Triangle3::uvs |
Three UV coordinates.
The documentation for this class was generated from the following file:
- Core/Geometry/Triangle3.hpp